Q1. An Employee Management System application is used to maintain information about employees in an organization. In the application, employee details are stored in the ascending order of the employee Ids. Which algorithmic design technique would best fit if an employee needs to be searched based on the employee ld.
A. Greedy Approach
B. Brute Force
C. Divide and Conquer
D. Dynamic Programming
Answer: A
Explanation:
Greedy algorithms are very fast. A lot faster than the two other alternatives (Divide & Conquer, and Dynamic Programming). They're used because they're fast. Most of the popular algorithms using Greedy have shown that Greedy gives the global optimal solution every time. Some of these include:
Dijkstra's Algorithm
Kruskal's algorithm
Prim's algorithm
Huffman trees
Q2. What is the output of the following code snippet?
public class Tester {
Public static void main (String[ ] args) {
for(int loop = 0;loop < 5;loop++) {
if(loop > 2) {
continue;
}
if(loop>4) {
break;
}
System.out.println(loop) ;
}
}
}
A:
0
1
2
B:
0
C:
0
1
2
3
4
D:
0
1
Answer: A
Explanation:
0th iteration:
loop<5 //True
loop>2→ Continue //False
loop>4→ Break //False
Print→ 0
1st iteration:
loop<5 //True
loop>2→ Continue //False
loop>4→ Break //False
Print→ 1
2nd iteration:
loop<5 //True
loop>2→ Continue //False
loop>4→ Break //False
Print→ 2
3rd iteration:
loop<5 //True
loop>2→ Continue //True
loop>4→ Break //no execution
Print→ //Nothing
4th iteration:
loop<5 //True
loop>2→ Continue //True
loop>4→ Break //no execution
Print→ //Nothing
Q3. Which of the following statements is TRUE with respect to Java language being platform independent?
A. The code in the java file is platform dependent
B. The JVM is the same across all operating systems
C. A java program written in a machine with Windows operating system cannot be executed on a machine having Linux operating system though Java is installed accordingly
D. A .class file can be run in any operating system where Java is installed
Answer: D
Explanation:
A. Java is a class-based and object-oriented programming language. It is a platform-independent language i.e. the compiled code can be run on any java supporting platform. It runs on the logic of “Write once, run anywhere”.
B. JVM makes java portable (write once, run anywhere). Each operating system has a different JVM, however, the output they produce after execution of byte code is the same across all operating systems.
C. The whole point of Java being platform-independent is for it to run in any operating system.
D. This is the only statement true.
Q4. What is the output of the following code snippet?
class Demo{
public static int specialAdd(int num1) {
if (num1!=0)
return (num1+2)+specialAdd(num1-1) ;
elsereturn 3;
}
public static int extraordinaryAdd(int num2) {
if (num2!=0)
return specialAdd(num2)+extraordinaryAdd(num2-1) ;
elsereturn 0;
}
public static void main (String [ ] args) {
System.out.println( (extraordinaryAdd(5) ) ) ;
}
}
A. 80
B. 52
C.70
D. 25
Answer: A
Explanation:
From this, we come to know that
specialAdd(5) = 28
specialAdd(4) = 21
specialAdd(3) = 15
specialAdd(2) = 10
specialAdd(1) = 6
specialAdd(0) = 3
Now to deal with extraordinaryAdd(4)
Therefore 28+52 = 80
Q5. What is the output of the code given below when run with the default Junit runner?
class Computation {
public int add(int num1, int num2) {
return num1 + num2 ;
}
public int divide(int num1, int num2) {
return num1 / num2 ;
}
}
public class TestComputation {
Computation comput = new Computation ( ) ;
@Test
public void testAdd1 ( ) {
int expected = 5 ;
int actual = comput.add(2, 3) ;
Assert.assertEquals(expected, actual) ;
}
@Test
public void testAdd2 ( ) {
int expected = 7 ;
int actual = comput.add(2, 5) ;
Assert.assertEquals(expected , actual) ;
}
}
A. Both testAdd1 and testAdd2 fail
B. testAdd1 fails and testAdd2 passes
C. Both testAdd1 and testAdd2 pass
D. testAdd1 passes and testAdd2 fails
Answer: C
Explanation:
Both would pass as expected and the actual values match in both
Q6. Consider the code snippet given below:
class Customer {
public int custId ;
public String custName ;
}
public class Tester {
public static void main (String args{ } ) {
Customer obj = new Customer ( ) ;
Customer objOne = new Customer ( ) ;
Customer objTwo ;
Customer objThree = obj ;
}
}
A. 3 objects and 1 reference variable
B. 2 objects and 4 reference variables
C. 4 objects and 4 reference variables
D. 2 objects and 3 reference variables
Answer: B
Explanation:
We know that an object is created by using a new keyword in java, a new keyword is used 2 times in the above code and hence 2 objects are created.
There are 4 references created in the above code namely→ obj, objOne, objTwo, objThree
Q7. Consider the code given below:
class Student {
private int studentId ;
private String studentName ;
Student (int studentId,String studentName) {
this.studentId = studentId ;
this.studentName = studentName ;
}
}
class College {
private Student studentId ;
private int basicFees ;
College (Student studentId, int basicFees) {
this.studentId = student ;
this.basicFees = basicFees ;
}
}
Identify the relationship between Student and College classes.
A. Aggregation
B. Association
C. Inheritance
D. The two classes are not related
Answer: A
Explanation:
Association is a semantically weak relationship (a semantic dependency) between otherwise unrelated objects. An association is a “using” relationship between two or more objects in which the objects have their own life and there is no owner.
An aggregation is a specialized form of association between two or more objects in which each object has its own life cycle but there exists ownership as well. Aggregation is a typical whole/part or parent/child relationship but it may or may not denote physical containment. An essential property of an aggregation relationship is that the whole or parent (i.e. the owner) can exist without the part or child and vice versa.
This code is an example of aggregation where class Student can exist without class College but not the other way around.
Q8. What is the output of the following code snippet?
public class ExceptionExample {
public void checkForExceptions(int num1, int num2) {
int intArr [ ] = {1,2,3} ;
String str = null ;
System.out.println("Before any exception!") ;
try{
str.charAt(0) ;
System.out.println(num1 / num1) ;
System.out.println("Enjoy no exception!") ;
}
catch (ArithmeticException e) {
System.out.println("ArithmeticException handler!") ;
} catch (NullPointerException e) {
System.out.println("NullPointException handler!") ;
} catch (Exception e) {
System.out.println("Default exception handler!") ;
} finally {
System.out.println("In finally!");
}
System.out.println("After handling exception!") ;
}
public static void main(String [ ] args)
{
ExceptionExample exceptionExample = new ExceptionExample( ) ;
try {
exceptionExample.checkForExceptions(2, 0) ;
} catch (ArithmeticException e) {
System.out.println("ArithmeticException handler in main!") ;
}
System.out.println("End of main") ;
}
}
A:
Before any exception!
Enjoy no exception!
In finally!
After handling exception!
End of main
B:
Before any exception!
Default exception handler!
In finally!
After handling exception!
End of main
C:
Before any exception!
ArithmeticException handler!
In finally!
After handling exception!
ArithmeticException handler in main!
End of main
D:
Before any exception!
NullPointerException handler!
In finally!
After handling exception!
End of main
Answer: D
Explanation:
So in this code, the execution starts from the driver code which has→ exceptionExample.checkForExceptions(2, 0) ; here the arguments are passed to the method checkForExceptions() which is present in the class. Before it starts the operation it prints “Before any exception” after which it executes str.charAt(0) ; this would throw an error as the str has been initialized with NULL. This exception would be caught and the statement would be printed accordingly as “NullPointException handler!”. As there are no further executions in the method checkForExceptions() control will return to the finally block, where “In finally!” will be printed. The final statement in the method “After handling exception!” will also be printed. The control returns to the driver code and the last line will be executed, that is to print “End of main”.
Q9.
Consider the problem size as ‘n’. Find the worst-case time complexity of the following algorithm.
if num1>num2 then
for (couter1=1;counter1<=n;counter1=counter1*2)
print(“num1 is greater than num2”)
end-for
else for(counter2=1;counter2<=n;counter2=counter2+1) {
print(“num2 is greater than num1”)
end-for
end-if
A. O(n)
B. O(n2)
C. O(log n)
D. O(n log n)
Answer: D
Explanation:
Time complexity of for (couter1=1;counter1<=n;counter1=counter1*2) would be O(log n)
Time complexity of for(counter2=1;counter2<=n;counter2=counter2+1) would be
O(n)
So the time complexity would be O(n log n)
Q10. Consider the code given below which is written in the file ‘Demo.java’.
class Book{
/ /Class definition
}
class Demo{
public static void main(String [ ] args) {
}
}
How many .class files will be generated for the above code and which class out of the two, Demo or Book, will be loaded into the main memory first when executed?
A. 2, Demo
B. 2, Book
C. 1, Demo
D. 1, Book
Answer: A
Explanation:
There are 2 classes here, class Book and class Demo, hence there will be 2 .class files created while the program gets compiled. Execution always starts from the class which has the main method, hence class Demo would be loaded first into the main memory.
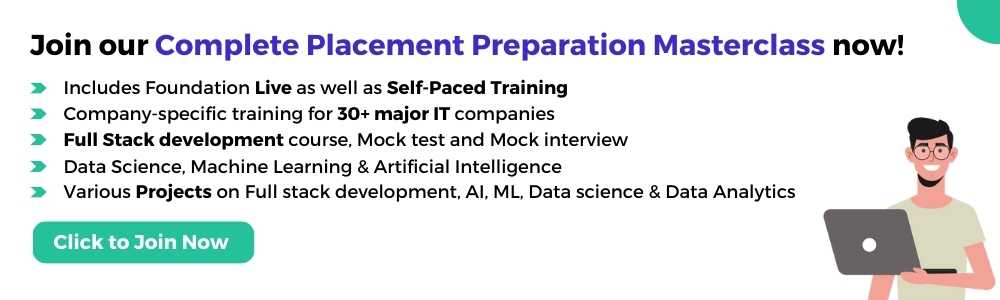
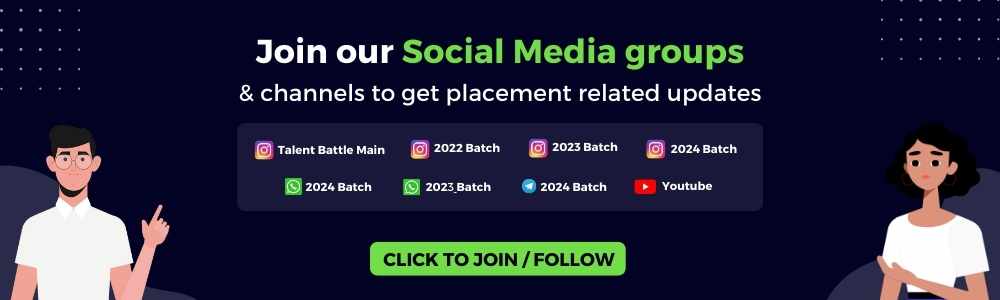