Data structures are essential components of computer science, enabling efficient data organization, storage, and manipulation. Various operations can be performed on data structures to manage and process data effectively. These operations can be broadly classified into the following categories:
1. Insertion
Insertion is the process of adding a new element to a data structure. The position of the new element can vary depending on the data structure type. For instance:
- Arrays: Elements can be inserted at any index, but this might require shifting existing elements.
- Linked Lists: Elements can be added at the beginning, end, or any specific position.
- Stacks: New elements are added to the top (Push operation).
- Queues: New elements are added to the rear (Enqueue operation).
- Trees: New nodes are added according to specific rules, such as in a binary search tree.
- Graphs: New vertices and edges can be added.
2. Deletion
Deletion is the process of removing an element from a data structure. Similar to insertion, the position of the element to be removed can vary:
- Arrays: Elements can be deleted from any index, which might require shifting remaining elements.
- Linked Lists: Nodes can be removed from the beginning, end, or a specific position.
- Stacks: The top element is removed (Pop operation).
- Queues: The front element is removed (Dequeue operation).
- Trees: Nodes are removed according to specific rules to maintain the tree's properties.
- Graphs: Vertices and edges can be removed.
3. Traversal
Traversal is the process of visiting all elements or nodes in a data structure in a specific order. Common traversal methods include:
- Arrays: Linear traversal from the first to the last element.
- Linked Lists: Sequential traversal from the head to the end node.
- Stacks: Accessing elements from top to bottom.
- Queues: Accessing elements from front to rear.
- Trees: Various traversal techniques like in-order, pre-order, post-order, and level-order.
- Graphs: Depth-first search (DFS) and breadth-first search (BFS).
4. Searching
Searching is the process of finding a specific element within a data structure. Techniques vary based on the structure:
- Arrays: Linear search or binary search (if the array is sorted).
- Linked Lists: Linear search from the head to the end node.
- Stacks and Queues: Typically involve linear search.
- Trees: Binary search (in binary search trees) or specific tree traversal methods.
- Graphs: DFS and BFS to locate specific vertices or edges.
5. Sorting
Sorting arranges the elements of a data structure in a specific order (ascending or descending). Common sorting algorithms include:
- Arrays: Quick sort, merge sort, bubble sort, and insertion sort.
- Linked Lists: Merge sort and quick sort (adapted for linked lists).
- Trees: In-order traversal of binary search trees results in sorted order.
6. Modification
Modification involves changing the value of an element within a data structure. This operation is straightforward in:
- Arrays: Direct access and update using the index.
- Linked Lists: Sequential access to the desired node, followed by value update.
- Stacks and Queues: Generally involves accessing elements through traversal.
7. Merging
Merging combines two data structures of the same type into one. This is commonly performed on:
- Arrays: Merging two sorted arrays into a single sorted array.
- Linked Lists: Combining two lists into one.
- Trees and Graphs: Combining nodes and edges while maintaining the properties of the data structure.
Conclusion
Understanding these operations is fundamental for efficiently managing and processing data using various data structures. Each operation has specific implications for performance and complexity, making it crucial to choose the appropriate data structure and operation based on the problem at hand. Whether it's inserting new elements, traversing through nodes, or merging data, mastering these operations enables programmers to build robust and efficient algorithms.
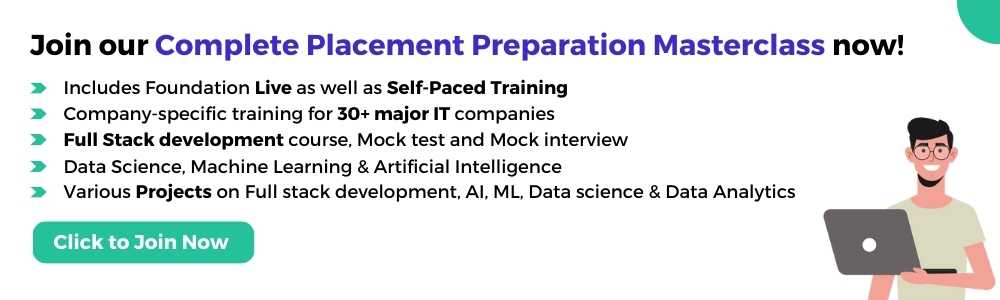
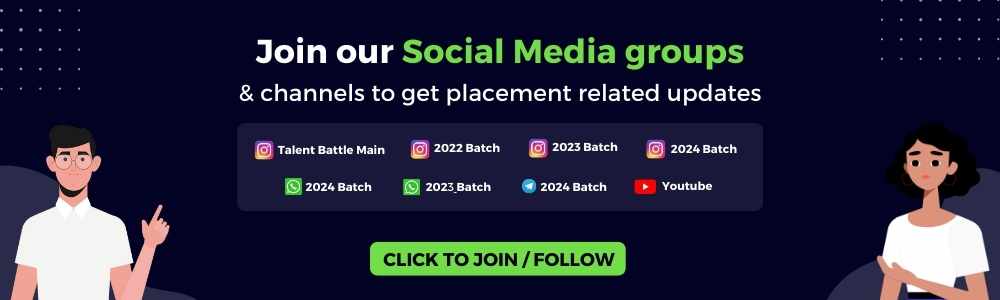