Data structures provide a way to organize and store data in a structured manner, establishing relationships between data elements for efficient processing. They form the foundation of many programming tools and methodologies.
Categories of Data Structures
Data structures are categorized into two main types:
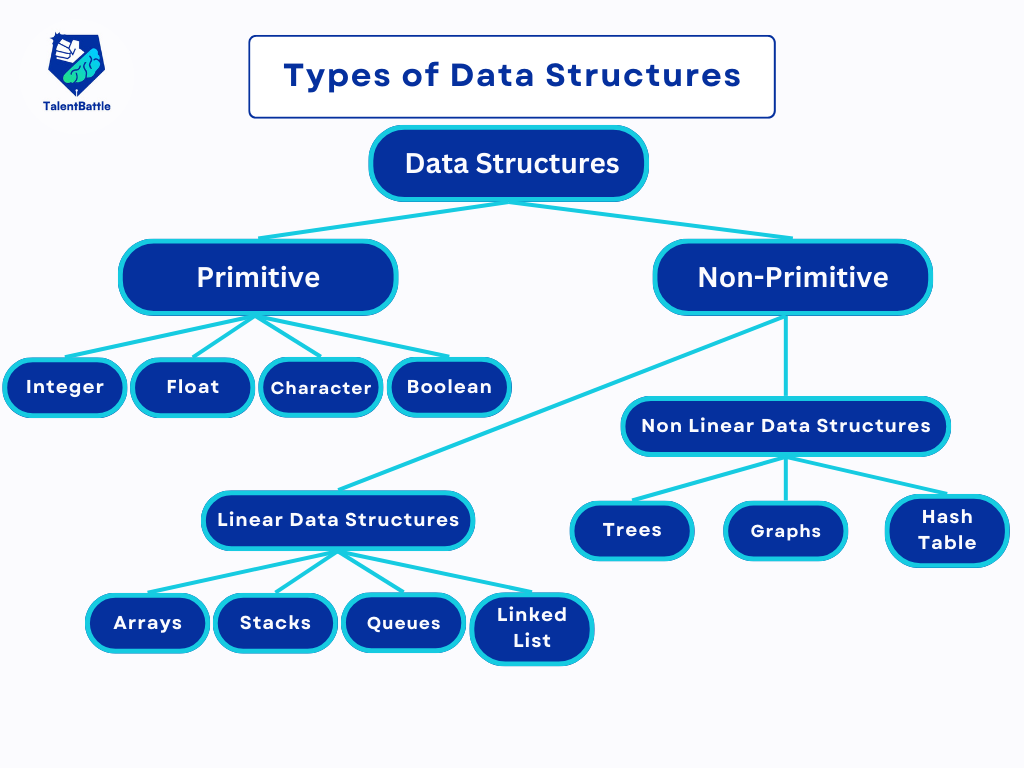
-
Primitive Data Structures: These include basic data types like integers, floats, characters, and booleans, which are built into the programming language and can be directly manipulated by machine-level instructions.
-
Non-Primitive Data Structures: These are derived from primitive data structures and focus on organizing a set of data elements that can be either homogeneous (same data type) or heterogeneous (different data types). They are not directly manipulated by machine-level instructions and are divided into two sub-categories:
-
Linear Data Structures: These maintain a sequential relationship among data elements, where each element is connected to its successor and predecessor, except for the first and last elements. Examples include arrays, linked lists, stacks, and queues.
-
Non-Linear Data Structures: In these structures, data elements are not arranged sequentially. Examples include trees and graphs, where elements are connected in a hierarchical or network manner.
Linear Data Structures
Static Data Structures
Static data structures have a fixed size, determined at compile time, and cannot be resized. Arrays are a common example, storing multiple data elements of the same type in contiguous memory locations.
Dynamic Data Structures
Dynamic data structures have a flexible size, determined at runtime, allowing the user to adjust their size and content during execution. Examples include linked lists, stacks, and queues.
Types of Linear Data Structures:
-
Arrays
- Arrays store multiple data elements of the same type in a single variable, with each element having a unique index. They can be one-dimensional, two-dimensional (matrices), or multidimensional.
Applications of Arrays:
- Store lists of data elements of the same type.
- Act as auxiliary storage for other data structures.
- Store data elements of a binary tree with a fixed count.
- Act as storage for matrices.
-
Linked Lists
- Linked lists store elements in nodes connected by pointers. Types include singly linked lists (each node points to the next), doubly linked lists (nodes have pointers to both previous and next nodes), and circular linked lists (last node points back to the first).
Applications of Linked Lists:
- Implement stacks, queues, binary trees, and graphs of predefined size.
- Implement dynamic memory management functions in operating systems.
- Allow polynomial implementation for mathematical operations.
- Implement round-robin execution of tasks in operating systems using circular linked lists.
- Implement forward and backward navigation in web browsers using doubly linked lists.
-
Stacks
- Stacks follow the LIFO (Last In, First Out) principle, allowing operations like insertion (push) and deletion (pop) only from the top of the stack. They can be implemented using arrays or linked lists.
Applications of Stacks:
- Serve as temporary storage for recursive operations.
- Act as auxiliary storage for function calls, nested operations, and deferred/postponed functions.
- Manage function calls.
- Evaluate arithmetic expressions in different programming languages.
- Convert infix expressions to postfix expressions.
- Check the syntax of expressions in a programming environment.
- Match parentheses in expressions.
- Reverse strings.
- Solve backtracking problems.
- Implement depth-first search in graph and tree traversal.
- Perform operating system functions.
- Implement UNDO and REDO functions in an editor.
-
Queues
- Queues follow the FIFO (First In, First Out) principle, with insertion (enqueue) at the rear and deletion (dequeue) at the front. They can be implemented using arrays, linked lists, or stacks.
Applications of Queues:
- Perform breadth-first search operations in graphs.
- Implement job scheduler operations in operating systems, like keyboard buffer queues and print buffer queues.
- Manage CPU scheduling, job scheduling, and disk scheduling.
- Implement priority queues for file downloading operations in browsers.
- Transfer data between peripheral devices and the CPU.
- Handle interrupts generated by user applications for the CPU.
Non-Linear Data Structures
Non-linear data structures do not arrange data elements sequentially. They establish hierarchical relationships among elements.
Types of Non-Linear Data Structures:
-
Trees
- Trees consist of nodes connected by edges, forming a hierarchy. Types include binary trees (each node has at most two children), binary search trees (maintain sorted order), AVL trees (self-balancing binary search trees), and B-trees (self-balancing trees with multiple keys per node).
Applications of Trees:
- Implement hierarchical structures in file systems.
- Implement website navigation structures.
- Generate codes like Huffman's code.
- Aid in decision-making in gaming applications.
- Implement priority queues for priority-based OS scheduling functions.
- Parse expressions and statements in compilers of different programming languages.
- Store data keys for indexing in Database Management Systems (DBMS).
- Route decisions in computer and communications networks using spanning trees.
- Implement path-finding algorithms in AI, robotics, and video games.
-
Graphs
- Graphs consist of vertices (nodes) connected by edges. Types include null graphs (no edges), simple graphs (no self-loops or multiple edges), directed graphs (edges have direction), connected graphs (at least one path between any two vertices), and more.
Applications of Graphs:
- Represent routes and networks in transportation, travel, and communication applications.
- Display routes in GPS systems.
- Represent interconnections in social networks and other network-based applications.
- Aid in mapping applications.
- Represent user preferences in e-commerce applications.
- Identify problems in utility networks for local or municipal corporations.
- Manage resource utilization and availability in organizations.
- Create document link maps of websites to display connectivity between pages through hyperlinks.
- Implement robotic motions and neural networks.
Applications of Data Structures
Arrays
Linked Lists
-
Useful for dynamic memory management, implementing stacks, queues, and trees, and performing polynomial operations.
Stacks
-
Employed in recursive operations, function calls, arithmetic expression evaluation, and syntax checking in programming languages.
Queues
Trees
-
Implement hierarchical structures in file systems, website navigation, decision-making in games, and indexing in databases.
Graphs
-
Represent routes and networks in transportation, social networks, mapping applications, and resource management in organizations.
By understanding and utilizing various data structures, programmers can efficiently manage and process data to meet specific application requirements.
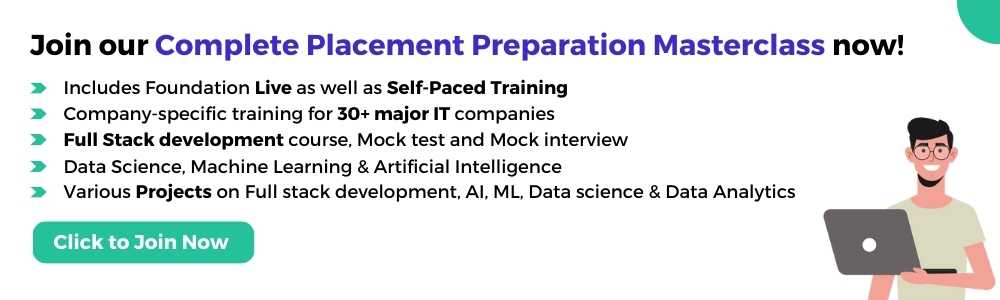
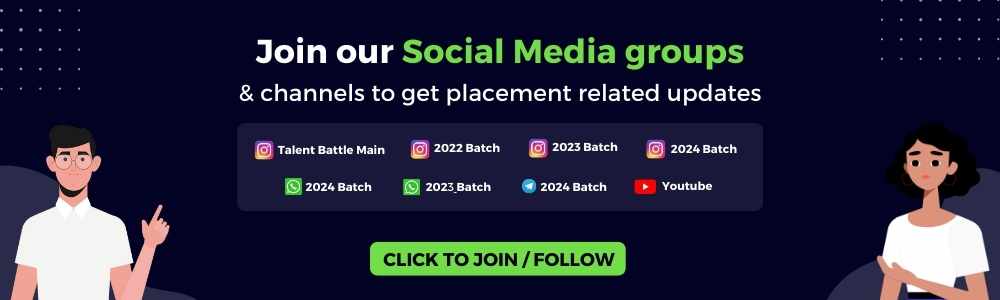