Trees are a widely used data structure that emulate a hierarchical tree structure with a set of connected nodes. Each node in a tree has a parent node and zero or more child nodes, making this structure ideal for representing hierarchical data.
.png)
Key Concepts of Trees
- Root: The top node of the tree, which has no parent.
- Node: An element in the tree that contains data.
- Edge: The link between two nodes.
- Leaf: A node with no children.
- Parent: A node that has one or more child nodes.
- Child: A node that has a parent node.
- Subtree: A tree consisting of a node and its descendants.
- Height: The length of the longest path from the root to a leaf.
- Depth: The length of the path from the root to a node.
.png)
Types of Trees
- Binary Tree: Each node has at most two children, referred to as the left child and the right child.
- Binary Search Tree (BST): A binary tree where the left child contains only nodes with values less than the parent node and the right child contains only nodes with values greater than the parent node.
- AVL Tree: A self-balancing binary search tree where the difference between the heights of left and right subtrees cannot be more than one for all nodes.
- Red-Black Tree: A self-balancing binary search tree where nodes are colored red or black to ensure the tree remains balanced.
- B-Tree: A self-balancing tree data structure that maintains sorted data and allows searches, sequential access, insertions, and deletions in logarithmic time.
- Heap: A specialized tree-based data structure that satisfies the heap property (max-heap or min-heap).
Basic Operations on Trees
- Insertion: Adding a node to the tree.
- Deletion: Removing a node from the tree.
- Traversal: Visiting all the nodes in the tree.
- Searching: Finding a node with a specific value.
Tree Traversal Methods
- In-order Traversal (LNR): Visit the left subtree, then the node, and finally the right subtree.
- Pre-order Traversal (NLR): Visit the node, then the left subtree, and finally the right subtree.
- Post-order Traversal (LRN): Visit the left subtree, then the right subtree, and finally the node.
- Level-order Traversal: Visit nodes level by level starting from the root.
Algorithms for Basic Operations
Insertion in a Binary Search Tree (BST)
Algorithm:
- Start from the root.
- Compare the value to be inserted with the value of the current node.
- If the value is less than the current node, go to the left subtree; if greater, go to the right subtree.
- Repeat the process until an appropriate position is found.
- Insert the new node at the position.
C++ Implementation:
struct Node {
int data;
Node* left;
Node* right;
Node(int value) : data(value), left(nullptr), right(nullptr) {}
};
Node* insert(Node* root, int key) {
if (root == nullptr) {
return new Node(key);
}
if (key < root->data) {
root->left = insert(root->left, key);
} else {
root->right = insert(root->right, key);
}
return root;
}
Deletion in a Binary Search Tree (BST)
Algorithm:
- Find the node to be deleted.
- If the node is a leaf, simply remove it.
- If the node has one child, replace the node with its child.
- If the node has two children, find the inorder successor (smallest in the right subtree), copy its value to the node, and delete the inorder successor.
C++ Implementation:
Node* findMin(Node* root) {
while (root->left != nullptr) {
root = root->left;
}
return root;
}
Node* deleteNode(Node* root, int key) {
if (root == nullptr) return root;
if (key < root->data) {
root->left = deleteNode(root->left, key);
} else if (key > root->data) {
root->right = deleteNode(root->right, key);
} else {
if (root->left == nullptr) {
Node* temp = root->right;
delete root;
return temp;
} else if (root->right == nullptr) {
Node* temp = root->left;
delete root;
return temp;
}
Node* temp = findMin(root->right);
root->data = temp->data;
root->right = deleteNode(root->right, temp->data);
}
return root;
}
In-order Traversal
Algorithm:
- Recursively visit the left subtree.
- Visit the node.
- Recursively visit the right subtree.
C++ Implementation:
void inOrderTraversal(Node* root) {
if (root == nullptr) return;
inOrderTraversal(root->left);
std::cout << root->data << " ";
inOrderTraversal(root->right);
}
Applications of Trees
- File System: Trees are used to represent the hierarchical structure of files and directories.
- Database Indexing: B-Trees and AVL Trees are used to implement database indexes, allowing efficient data retrieval.
- Network Routing: Trees are used in network routing algorithms to find the shortest path.
- XML/HTML Parsing: Trees are used to represent the structure of XML and HTML documents for parsing.
- Game Development: Trees are used in AI for decision-making, such as in game trees.
Conclusion
Trees are a fundamental data structure in computer science, providing an efficient way to store and manipulate hierarchical data. Understanding the various types of trees and their operations is crucial for solving many computational problems.
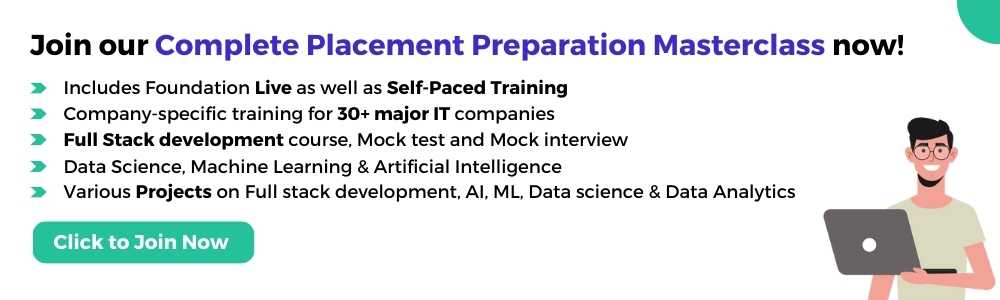
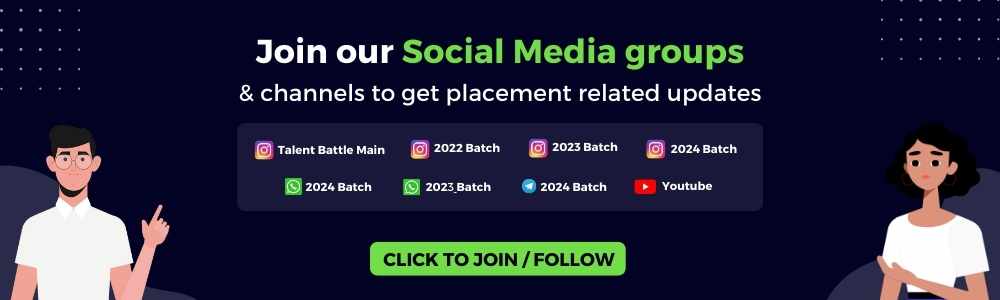