Table of Contents (Click any Topic to view first)
- Abstract Classes
- Interfaces
- Abstract Class vs Interface
- Advantages of Abstraction
- Real-World Example
Abstraction in Java is a process of hiding the implementation details from the user and only providing the functionality. In Java, abstraction is achieved using abstract classes and interfaces.
An abstract class is a class that cannot be instantiated. It is used to declare common characteristics for all subclasses. Abstract classes can have abstract methods (methods without a body) as well as concrete methods (methods with a body).
Key Points:
- An abstract class cannot be instantiated.
- It can have abstract and non-abstract methods.
- It can have constructors and static methods.
- It can have final methods which will force the subclass not to change the body of the method.
Example:
abstract class Animal {
// Abstract method (does not have a body)
abstract void makeSound();
// Regular method
void eat() {
System.out.println("This animal eats food.");
}
}
class Dog extends Animal {
// Providing implementation for the abstract method
void makeSound() {
System.out.println("Bark");
}
public static void main(String[] args) {
Dog d = new Dog();
d.makeSound();
d.eat();
}
}
An interface in Java is a blueprint of a class. It has static constants and abstract methods. Interfaces are used to achieve full abstraction.
Key Points:
- An interface cannot have instance variables.
- All the methods in an interface are abstract by default.
- Interfaces support multiple inheritance.
- An interface can extend multiple interfaces.
- A class can implement multiple interfaces.
Example:
interface Animal {
// Abstract method
void makeSound();
// Default method
default void eat() {
System.out.println("This animal eats food.");
}
}
class Dog implements Animal {
// Providing implementation for the abstract method
public void makeSound() {
System.out.println("Bark");
}
public static void main(String[] args) {
Dog d = new Dog();
d.makeSound();
d.eat();
}
}
Feature
|
Abstract Class
|
Interface
|
Method Implementation
|
Can have both abstract and concrete methods
|
Only abstract methods (Java 8 allows default methods)
|
Inheritance
|
Can extend only one class
|
Can implement multiple interfaces
|
Constructors
|
Can have constructors
|
Cannot have constructors
|
Fields
|
Can have instance variables
|
Can only have static final variables (constants)
|
Access Modifiers
|
Can have any access modifiers
|
Methods are implicitly public abstract, fields are public static final
|
- Simplifies Code: By hiding the complex implementation details and showing only the necessary parts, abstraction helps in simplifying the code.
- Enhances Security: Only essential information is provided, hiding the internal details and thereby enhancing security.
- Improves Maintainability: With abstraction, the code becomes more manageable and easier to understand, thus improving maintainability.
- Promotes Reusability: Abstract classes and interfaces can be reused across different parts of the application, reducing code duplication.
Consider a system where you need to represent different types of vehicles (e.g., Car, Bike). Both vehicles share some common behavior (e.g., startEngine, stopEngine), but also have their specific behavior. Abstract classes and interfaces can be used to define a common structure and provide specific implementations.
Example:
abstract class Vehicle {
abstract void startEngine();
abstract void stopEngine();
void fuel() {
System.out.println("Refueling vehicle...");
}
}
class Car extends Vehicle {
void startEngine() {
System.out.println("Car engine started.");
}
void stopEngine() {
System.out.println("Car engine stopped.");
}
}
class Bike extends Vehicle {
void startEngine() {
System.out.println("Bike engine started.");
}
void stopEngine() {
System.out.println("Bike engine stopped.");
}
}
public class Test {
public static void main(String[] args) {
Vehicle myCar = new Car();
myCar.startEngine();
myCar.fuel();
myCar.stopEngine();
Vehicle myBike = new Bike();
myBike.startEngine();
myBike.fuel();
myBike.stopEngine();
}
}
Conclusion
Abstraction in Java helps to reduce complexity and allows focusing on what an object does instead of how it does it. By using abstract classes and interfaces, you can create a flexible and scalable codebase that is easier to maintain and extend.
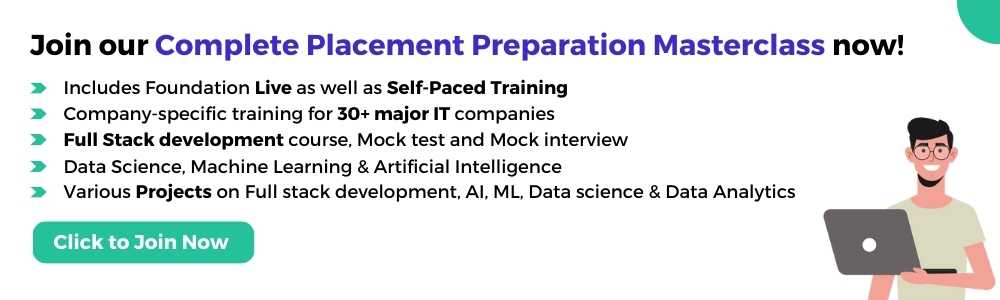
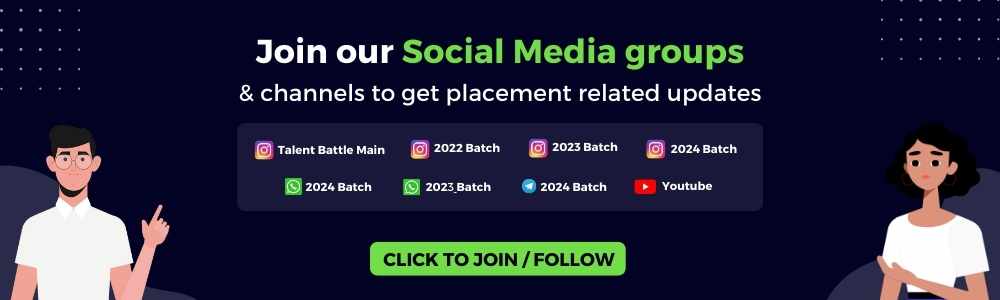