Table of Contents (Click any Topic to view first)
- Static Binding (Early Binding)
- Dynamic Binding (Late Binding)
- Understanding Type in Java
Binding in Java refers to the process of associating a method call with the method body. There are two types of binding:
- Static Binding (Early Binding)
- Dynamic Binding (Late Binding)
Static binding occurs when the type of the object is determined at compile-time by the compiler. This type of binding is used for:
- Private methods
- Final methods
- Static methods
Since these methods cannot be overridden, the method call is resolved at compile-time.
Example:
class Dog {
private void eat() {
System.out.println("dog is eating...");
}
public static void main(String[] args) {
Dog d1 = new Dog();
d1.eat();
}
}
Explanation: In the above example, the eat method is private, so the compiler knows exactly which method to call at compile-time, resulting in static binding.
Dynamic Binding (Late Binding)
Dynamic binding occurs when the type of the object is determined at runtime. This happens when the method is overridden in a subclass.
Example:
class Animal {
void eat() {
System.out.println("animal is eating...");
}
}
class Dog extends Animal {
void eat() {
System.out.println("dog is eating...");
}
public static void main(String[] args) {
Animal a = new Dog();
a.eat();
}
}
Explanation: In the above example, the eat method is overridden in the Dog class. The reference variable a is of type Animal, but it holds an instance of Dog. At runtime, the JVM determines the actual object type and calls the eat method of the Dog class, resulting in dynamic binding.
- Variables Have a Type:
int data = 30; // Here, data is of type int
- References Have a Type:
class Dog {
public static void main(String[] args) {
Dog d1; // Here, d1 is a reference of type Dog
}
}
- Objects Have a Type: An object is an instance of a particular class and can also be considered an instance of its superclass.
class Animal {}
class Dog extends Animal {
public static void main(String[] args) {
Dog d1 = new Dog();
// d1 is an instance of Dog, which is also an instance of Animal
}
}
Summary
- Static Binding: Determined at compile-time. Used for private, final, and static methods.
- Dynamic Binding: Determined at runtime. Used for overridden methods.
Binding plays a crucial role in polymorphism, where dynamic binding allows Java to achieve runtime polymorphism, enabling flexible and reusable code.
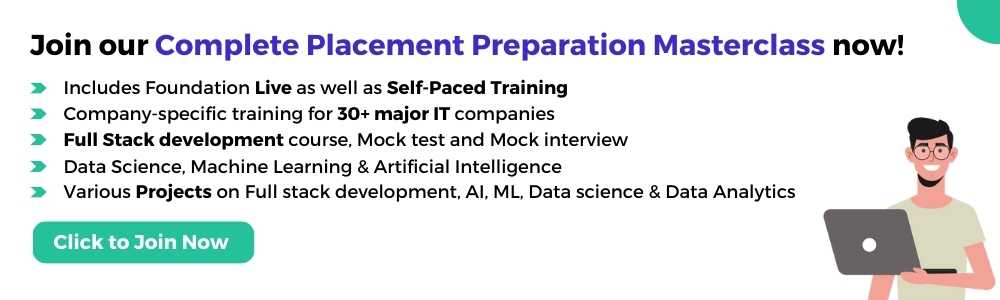
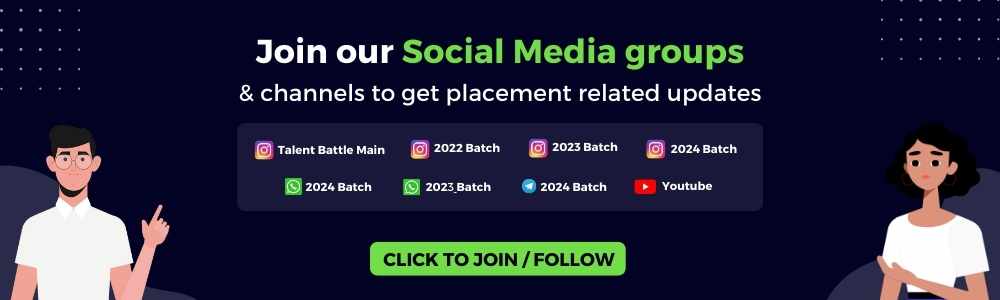