Table of Contents (Click any Topic to view first)
- Syntax of the Break Statement
- Example 1: Using Break in a for Loop
- Example 2: Using Break in a while Loop
- Example 3: Using Break in a switch Statement
Understanding the Break Statement in Java
In Java programming, the break
statement provides a mechanism to prematurely exit a loop or switch statement. It's particularly useful for terminating loop execution based on certain conditions or when a specific task is completed. Let's explore the break
statement in Java with detailed explanations and examples.
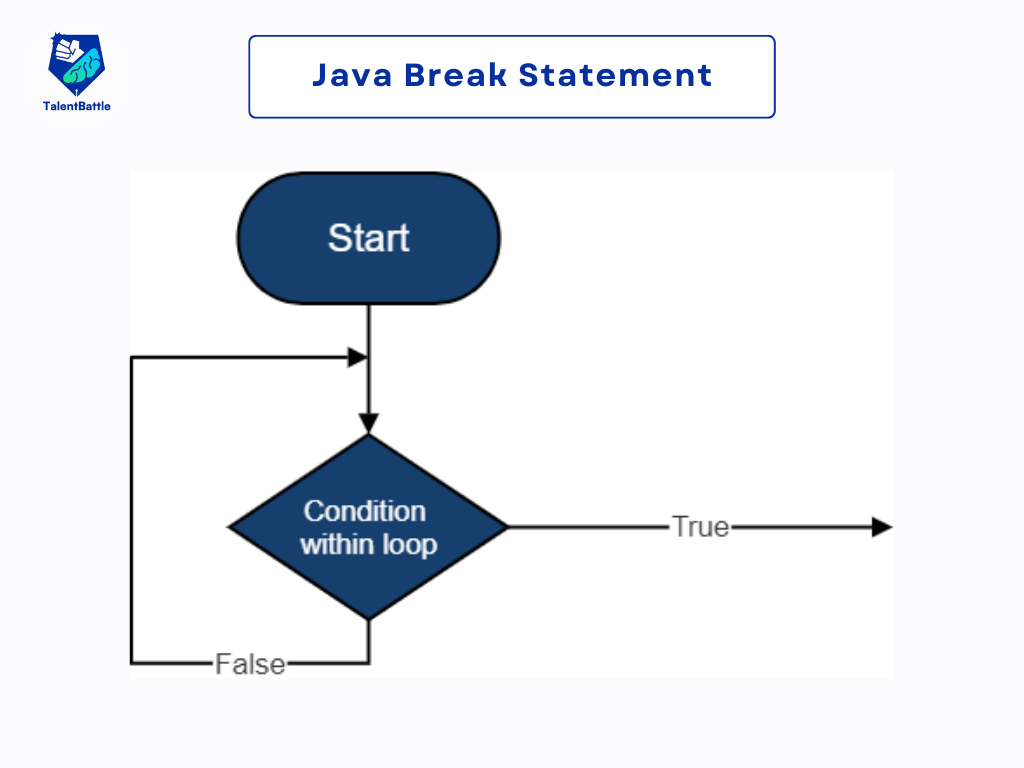
The break
statement is simple in its syntax and usage:
break;
When encountered inside a loop or switch statement, the break
statement immediately terminates the loop or switch, and the program continues with the statement following the loop or switch.
public class BreakInForLoop {
public static void main(String[] args) {
for (int i = 1; i <= 5; i++) {
System.out.println("Iteration " + i);
if (i == 3) {
break; // Exit the loop when i is 3
}
}
System.out.println("Loop exited");
}
}
In this example, the for
loop iterates from 1 to 5, printing each iteration number. However, when i
becomes 3, the break
statement is encountered, causing the loop to terminate prematurely. As a result, the program prints "Loop exited" after exiting the loop.
public class BreakInWhileLoop {
public static void main(String[] args) {
int i = 1;
while (i <= 5) {
System.out.println("Iteration " + i);
if (i == 3) {
break; // Exit the loop when i is 3
}
i++;
}
System.out.println("Loop exited");
}
}
Similar to the previous example, this code demonstrates the use of the break
statement within a while
loop. The loop exits when i
equals 3, and the program prints "Loop exited" afterward.
public class BreakInSwitchStatement {
public static void main(String[] args) {
int day = 4;
switch (day) {
case 1:
System.out.println("Sunday");
break;
case 2:
System.out.println("Monday");
break;
case 3:
System.out.println("Tuesday");
break;
default:
System.out.println("Unknown day");
break;
}
}
}
In this example, the break
statement is used within a switch
statement to exit the switch block after executing the corresponding case. Once the appropriate case is found and executed, the break
statement ensures that the switch block is exited, preventing fall-through to subsequent cases.
Conclusion:
The break
statement in Java provides a means to control loop and switch execution flow by prematurely exiting the enclosing loop or switch. Whether used in for
loops, while
loops, or switch
statements, the break
statement offers flexibility and control in program flow.
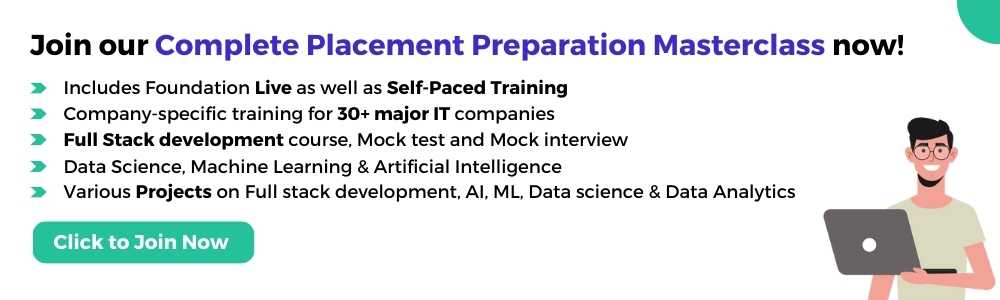
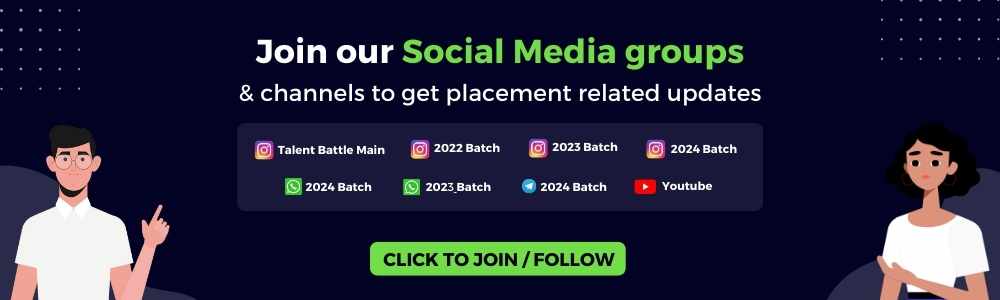