Table of Contents (Click any Topic to view first)
- Classes in Java
- Objects in Java
- Example: Creating a Class and Object in Java
- Key Concepts
Understanding the Classes and Objects (OOP's Concepts) in Java
In Java programming, objects and classes form the backbone of object-oriented programming (OOP) principles. They enable developers to model real-world entities and encapsulate their behavior and state. Let's explore objects and classes in Java in detail, along with examples to illustrate their usage.
- Definition: A class in Java is a blueprint or template that defines the structure and behavior of objects. It encapsulates data (attributes) and methods (functions) to operate on that data.
- Syntax:
public class ClassName {
// Data members (fields)
// Constructor(s)
// Methods
}
Objects in Java:
- Definition: An object is an instance of a class. It represents a specific entity with its unique state (attributes) and behavior (methods).
- Syntax:
ClassName objectName = new ClassName();
// Class definition
public class Car {
// Data members
String brand;
String model;
int year;
// Constructor
public Car(String brand, String model, int year) {
this.brand = brand;
this.model = model;
this.year = year;
}
// Method to display car details
public void displayDetails() {
System.out.println("Brand: " + brand);
System.out.println("Model: " + model);
System.out.println("Year: " + year);
}
}
// Main class
public class Main {
public static void main(String[] args) {
// Creating an object of the Car class
Car myCar = new Car("Toyota", "Camry", 2021);
// Accessing object's methods
myCar.displayDetails();
}
}
In this example:
- We define a Car class with data members brand, model, and year, along with a constructor to initialize these attributes.
- We create an object myCar of the Car class using the new keyword.
- We access the displayDetails() method of the myCar object to print its details.
Key Concepts:
- Class: Defines the blueprint or template for objects.
- Object: Represents a specific instance of a class with its unique state and behavior.
- Encapsulation: Bundles data and methods within a class, hiding the internal implementation details.
- Constructor: Initializes the state of an object when it is created.
- Method: Defines behaviors or actions that objects can perform.
Conclusion:
Understanding objects and classes is fundamental in Java programming. By defining classes and creating objects, developers can model complex systems, organize code logically, and build scalable and maintainable applications.
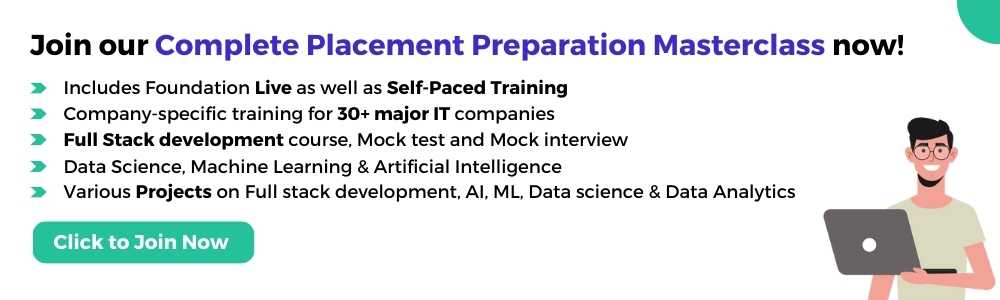
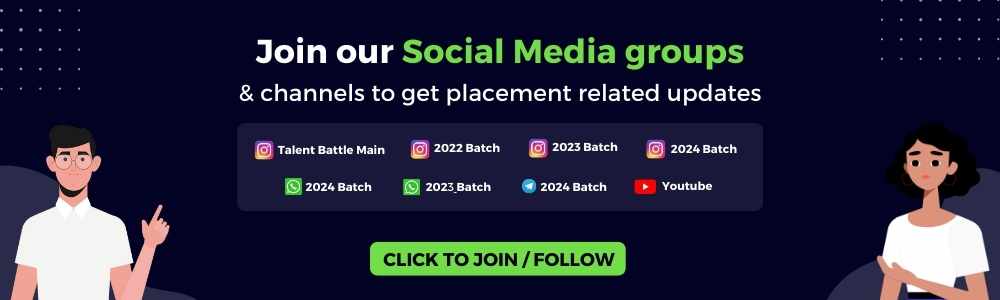