Table of Contents (Click any Topic to view first)
- Introduction to Collections (List, Set, Map)
- Iteration and Manipulation of Collections
- Generics in Collections
The Java Collections Framework provides a set of interfaces and classes for storing and manipulating groups of data as a single unit. This framework is part of the java.util
package and includes various classes such as ArrayList
, HashSet
, HashMap
, etc.
List
A List
is an ordered collection that can contain duplicate elements. Elements in a list can be accessed by their position (index).
Example:
import java.util.ArrayList;
import java.util.List;
public class ListExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
list.add("Apple"); // Duplicate element
for (String fruit : list) {
System.out.println(fruit);
}
}
}
Set
A Set
is a collection that cannot contain duplicate elements. It models the mathematical set abstraction.
-
HashSet: Implements the Set
interface, backed by a hash table (actually a HashMap
instance).
-
LinkedHashSet: Hash table and linked list implementation of the Set
interface, with predictable iteration order.
-
TreeSet: Implements the NavigableSet
interface, backed by a TreeMap
. The elements are ordered using their natural ordering or a comparator.
Example:
import java.util.HashSet;
import java.util.Set;
public class SetExample {
public static void main(String[] args) {
Set<String> set = new HashSet<>();
set.add("Apple");
set.add("Banana");
set.add("Cherry");
set.add("Apple"); // Duplicate element, will not be added
for (String fruit : set) {
System.out.println(fruit);
}
}
}
Map
A Map
is an object that maps keys to values. A map cannot contain duplicate keys, and each key can map to at most one value.
-
HashMap: Hash table-based implementation of the Map
interface.
-
LinkedHashMap: Hash table and linked list implementation of the Map
interface, with predictable iteration order.
-
TreeMap: Red-Black tree-based implementation of the NavigableMap
interface.
Example:
import java.util.HashMap;
import java.util.Map;
public class MapExample {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("Apple", 1);
map.put("Banana", 2);
map.put("Cherry", 3);
map.put("Apple", 4); // Duplicate key, will overwrite the previous value
for (Map.Entry<String, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
Iteration
There are several ways to iterate over collections in Java:
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
for (String fruit : list) {
System.out.println(fruit);
}
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class IteratorExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String fruit = iterator.next();
if ("Banana".equals(fruit)) {
iterator.remove(); // Remove element during iteration
}
System.out.println(fruit);
}
}
}
import java.util.ArrayList;
import java.util.List;
import java.util.ListIterator;
public class ListIteratorExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
ListIterator<String> listIterator = list.listIterator();
while (listIterator.hasNext()) {
String fruit = listIterator.next();
if ("Banana".equals(fruit)) {
listIterator.set("Blueberry"); // Modify element
}
}
for (String fruit : list) {
System.out.println(fruit);
}
}
}
Manipulation
Common manipulation operations include adding, removing, and updating elements.
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
Set<String> set = new HashSet<>();
set.add("Apple");
set.add("Banana");
Map<String, Integer> map = new HashMap<>();
map.put("Apple", 1);
map.put("Banana", 2);
list.remove("Banana");
set.remove("Banana");
map.remove("Banana");
list.set(1, "Blueberry"); // Update element at index 1
map.put("Apple", 4); // Update value for key "Apple"
Generics provide a way to define classes, interfaces, and methods with a placeholder for types (parameters) to ensure type safety.
Example of Generics in Collections:
import java.util.ArrayList;
import java.util.List;
public class GenericsExample {
public static void main(String[] args) {
// Using generics to ensure type safety
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
// list.add(123); // Compile-time error
for (String fruit : list) {
System.out.println(fruit);
}
}
}
Advantages of Generics
-
Type Safety: Ensures that you can only add the specified type to the collection, reducing runtime errors.
-
Elimination of Casts: Reduces the need for casting when retrieving elements from a collection.
// Without generics
List list = new ArrayList();
list.add("Apple");
String fruit = (String) list.get(0); // Need to cast
// With generics
List<String> genericList = new ArrayList<>();
genericList.add("Apple");
String genericFruit = genericList.get(0); // No cast needed
-
Enhanced Code Reusability: Allows for the creation of classes, interfaces, and methods that can be reused for different data types.
Generic Methods
Methods can also be generic.
public class GenericMethodExample {
// Generic method
public static <T> void printArray(T[] array) {
for (T element : array) {
System.out.println(element);
}
}
public static void main(String[] args) {
Integer[] intArray = {1, 2, 3, 4, 5};
String[] stringArray = {"Apple", "Banana", "Cherry"};
printArray(intArray); // Calling generic method with Integer array
printArray(stringArray); // Calling generic method with String array
}
}
Conclusion
The Java Collections Framework provides a comprehensive set of interfaces and classes for handling groups of objects. By understanding and utilizing lists, sets, maps, iteration techniques, and generics, you can write more efficient, type-safe, and maintainable Java Programs.
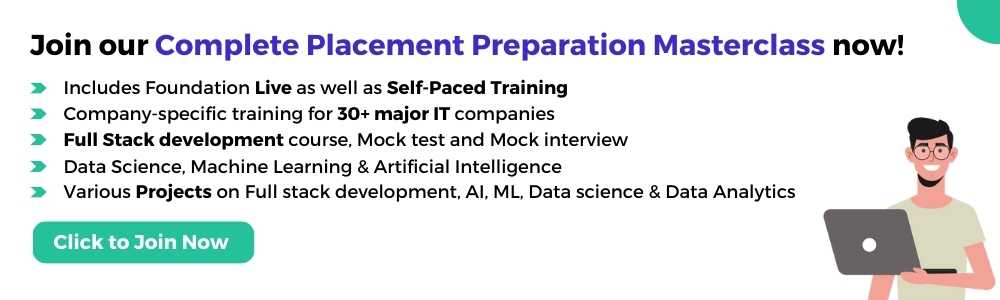
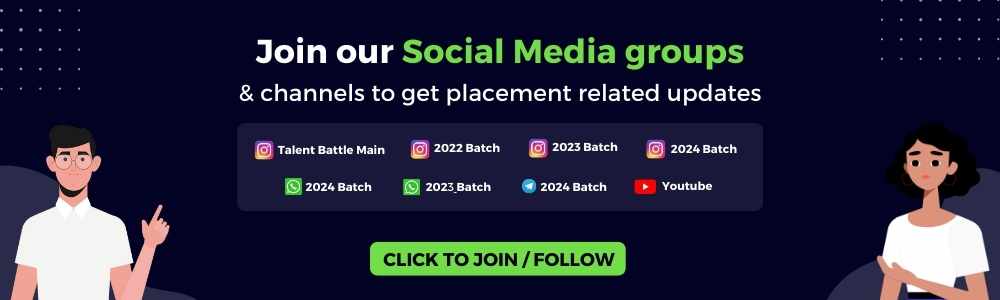