Table of Contents (Click any Topic to view first)
- What are Comments in Java?
- Why are Comments Important?
- Types of Comments in Java
- Best Practices for Writing Comments
Are you new to Java programming and wondering what those lines starting with // or /* are in your code? Don't worry; they're comments! Let's dive into the world of Java comments and understand how they work.
In Java, comments are lines of text added to the code to explain what the code does. These comments are ignored by the compiler, meaning they don't affect how the program runs. They are solely for the benefit of developers to understand the code better.
Comments play a crucial role in programming for several reasons:
- Code Explanation: Comments help explain the purpose of variables, methods, or complex algorithms, making it easier for developers to understand the code, especially when revisiting it after some time.
- Documentation: Comments can serve as documentation for other developers who may work on the codebase in the future. Good documentation helps maintain and update code effectively.
- Debugging: Comments can be used to temporarily disable lines of code during debugging without deleting them. This practice can be handy when troubleshooting issues.
Java supports three types of comments:
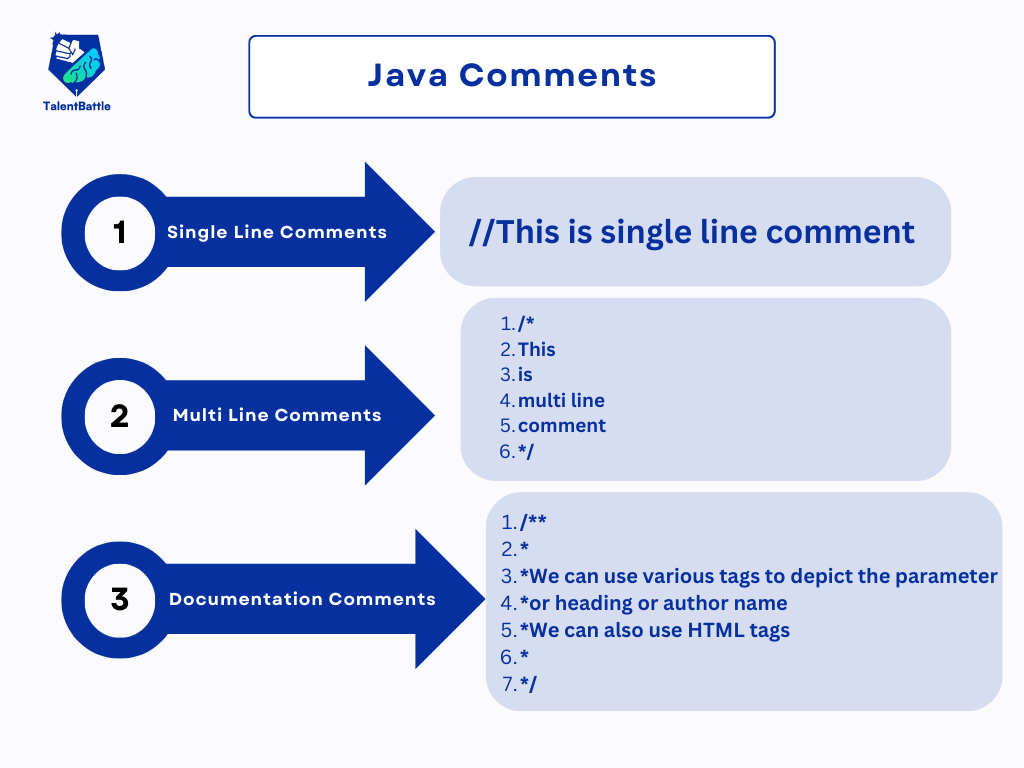
- Single-Line Comments: These comments start with // and extend to the end of the line. They are ideal for short explanations or notes on a single line of code.
Example:
// This is a single-line comment
int x = 10; // Initialize variable x with value 10
- Multiline Comments: Multiline comments begin with /* and end with */. They can span multiple lines and are useful for commenting out large blocks of code or providing detailed explanations.
Example:
/*
* This is a multiline comment.
* It can span multiple lines.
*/
int y = 20;
- Documentation Comments: These comments start with /** and end with */. They are specifically formatted for generating documentation using tools like Javadoc. Documentation comments provide information about classes, methods, parameters, return values, and exceptions.
Example:
/**
* This method divides two numbers.
*
* @param dividend The number to be divided
* @param divisor The number by which the dividend is divided
* @return The result of the division
* @throws IllegalArgumentException if divisor is zero
*/
public double divide(int dividend, int divisor) throws IllegalArgumentException {
// Code implementation here
}
- Be Clear and Concise: Write comments that are easy to understand and get straight to the point.
- Update Comments: Keep comments up-to-date with code changes to ensure they remain accurate and helpful.
- Avoid Redundancy: Don't over-comment; focus on adding value where necessary.
- Use Descriptive Names: Use meaningful variable and method names to reduce the need for excessive comments.
- Follow Style Guidelines: Adhere to coding standards and conventions for consistent comment formatting across the codebase.
In conclusion, comments are an essential part of Java programming for enhancing code readability, facilitating collaboration, and ensuring maintainability. By understanding and using comments effectively, developers can write cleaner, more understandable code. So, don't hesitate to add comments to your Java code and make your programming journey smoother!
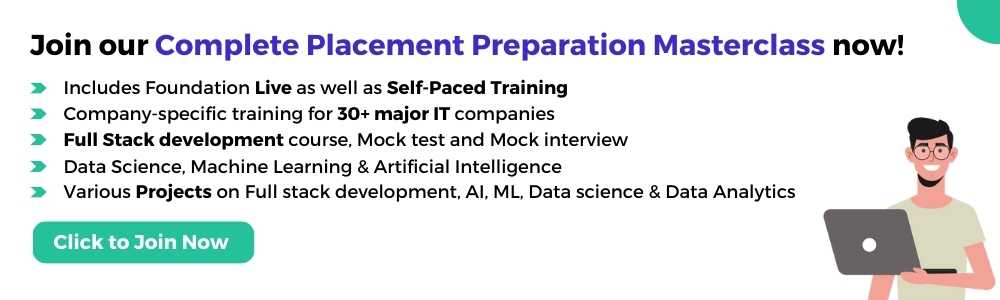
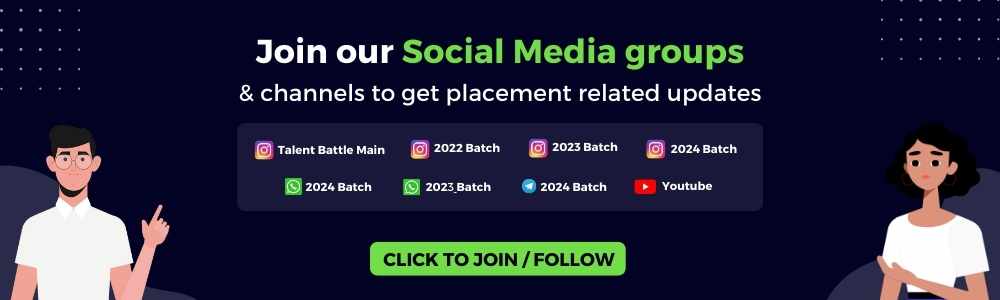