Table of Contents (Click any Topic to view first)
- Syntax of the Continue Statement
- Example 1: Using Continue in a for Loop
- Example 2: Using Continue in a while Loop
- Example 3: Using Continue in a Nested Loop
Understanding the Continue Statement in Java
In Java programming, the continue
statement offers a way to skip the current iteration of a loop and proceed directly to the next iteration. It's particularly useful for controlling loop execution flow when certain conditions are met within an iteration. Let's explore the continue
statement in Java with detailed explanations and examples.
The continue
statement is straightforward in its syntax and usage:
continue;
When encountered inside a loop, the continue
statement causes the current iteration to be skipped, and the loop proceeds with the next iteration.
public class ContinueInForLoop {
public static void main(String[] args) {
for (int i = 1; i <= 5; i++) {
if (i == 3) {
continue; // Skip iteration when i is 3
}
System.out.println("Iteration " + i);
}
}
}
In this example, the for
loop iterates from 1 to 5, but when i
equals 3, the continue
statement is encountered. As a result, the iteration for i = 3
is skipped, and the loop proceeds with the next iteration. The output will exclude "Iteration 3".
public
class
ContinueInWhileLoop {
public static void main(String[] args) {
int i = 1;
while (i <= 5) {
if (i == 3) {
i++; // Increment i to avoid infinite loop
continue; // Skip iteration when i is 3
}
System.out.println("Iteration " + i);
i++;
}
}
}
Similarly, this code demonstrates the use of the continue
statement within a while
loop. When i
equals 3, the current iteration is skipped using continue
, and the loop proceeds with the next iteration.
public class ContinueInNestedLoop {
public static void main(String[] args) {
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
if (j == 2) {
continue; // Skip inner loop when j is 2
}
System.out.println("i: " + i + ", j: " + j);
}
}
}
}
In this example, the continue
statement is used within a nested loop structure. When j
equals 2 in the inner loop, the continue
statement skips the rest of the inner loop iterations for that specific iteration of the outer loop.
Conclusion:
The continue
statement in Java provides a mechanism to control loop execution flow by skipping the current iteration when certain conditions are met. Whether used in for
loops, while
loops, or nested loops, the continue
statement offers precision and control in program flow.
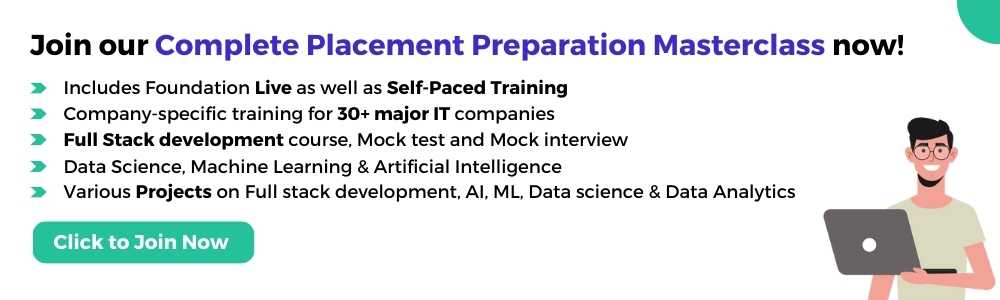
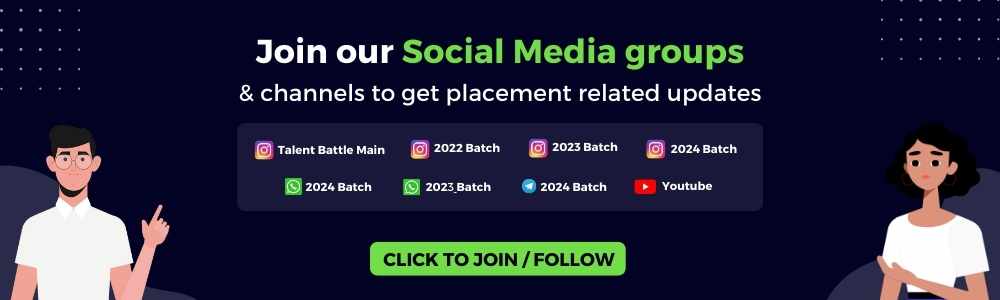