Table of Contents (Click any Topic to view first)
- Decision-Making Statements
- Loop Statements
- Jump Statements
In Java programming, the compiler typically executes code from top to bottom, following the sequence in which statements are written. However, Java offers control flow statements that enable programmers to control the execution flow of their code. These statements are known as control flow statements and are fundamental to Java programming.
.png)
Decision-making statements determine which statement to execute based on specific conditions. In Java, there are two main types of decision-making statements: if statements and switch statements.
- If Statement: The if statement evaluates a condition and executes a block of code if the condition is true.
Syntax:
if (condition) {
// Code block executed if condition is true
}
Example:
public class IfExample {
public static void main(String[] args) {
int x = 10;
int y = 12;
if (x + y > 20) {
System.out.println("x + y is greater than 20");
}
}
}
- Switch Statement: The switch statement evaluates an expression and executes code blocks based on different values of the expression.
Syntax:
switch (expression) {
case value1:
// Code block executed if expression equals value1
break;
// Other cases...
default:
// Code block executed if expression doesn't match any case
}
Example:
public class SwitchExample {
public static void main(String[] args) {
int day = 2;
String dayName;
switch (day) {
case 1:
dayName = "Monday";
break;
// Other cases...
default:
dayName = "Unknown";
}
System.out.println("Day is: " + dayName);
}
}
Loop statements allow you to repeat a block of code multiple times. Java provides several loop statements: for, while, do-while, and for-each.
- For Loop: The for loop executes a block of code a specified number of times.
Syntax:
for (initialization; condition; increment/decrement) {
// Code block to be executed
}
Example:
public class ForLoopExample {
public static void main(String[] args) {
int sum = 0;
for (int j = 1; j <= 10; j++) {
sum = sum + j;
}
System.out.println("The sum of first 10 natural numbers is " + sum);
}
}
- While Loop: The while loop repeats a block of code as long as a condition is true.
Syntax:
while (condition) {
// Code block to be executed
}
Example:
public class WhileLoopExample {
public static void main(String[] args) {
int i = 0;
System.out.println("Printing the list of first 10 even numbers \n");
while (i <= 10) {
System.out.println(i);
i = i + 2;
}
}
}
- Do-While Loop: The do-while loop is similar to the while loop, but it guarantees at least one execution of the code block before checking the condition.
Syntax:
do {
// Code block to be executed
} while (condition);
Example:
public class DoWhileLoopExample {
public static void main(String[] args) {
int i = 0;
System.out.println("Printing the list of first 10 even numbers \n");
do {
System.out.println(i);
i = i + 2;
} while (i <= 10);
}
}
- For-Each Loop: The for-each loop is used to iterate over elements in an array or collection.
Synatx:
for (datatype variable : array/collection) {
// Code block to be executed
}
Example:
public class ForEachLoopExample {
public static void main(String[] args) {
String[] names = {"Java", "C", "C++", "Python", "JavaScript"};
System.out.println("Printing the content of the array names:\n");
for (String name : names) {
System.out.println(name);
}
}
}
Jump Statements:
Jump statements alter the normal flow of control in a Java program. They include the break and continue statements.
- Break Statement: The break statement terminates the loop or switch statement and transfers control to the statement immediately following the loop or switch.
Syntax:
break;
Example:
public class BreakExample {
public static void main(String[] args) {
for (int i = 0; i <= 10; i++) {
System.out.println(i);
if (i == 6) {
break;
}
}
}
}
- Continue Statement: The continue statement skips the current iteration of a loop and proceeds to the next iteration.
Syntax:
continue;
Example:
public class ContinueExample {
public static void main(String[] args) {
for (int i = 0; i <= 2; i++) {
for (int j = i; j <= 5; j++) {
if (j == 4) {
continue;
}
System.out.println(j);
}
}
}
}
These control flow statements are crucial tools for Java programmers to manage the flow of execution in their programs efficiently. By understanding and using them effectively, programmers can create more flexible and dynamic Java applications.
In Java programming, decision-making statements allow you to control the flow of your program based on specific conditions. One of the fundamental decision-making statements in Java is the if-else statement. This statement evaluates a condition and executes different blocks of code depending on whether the condition is true or false. Let's explore the if-else statement in detail with examples.
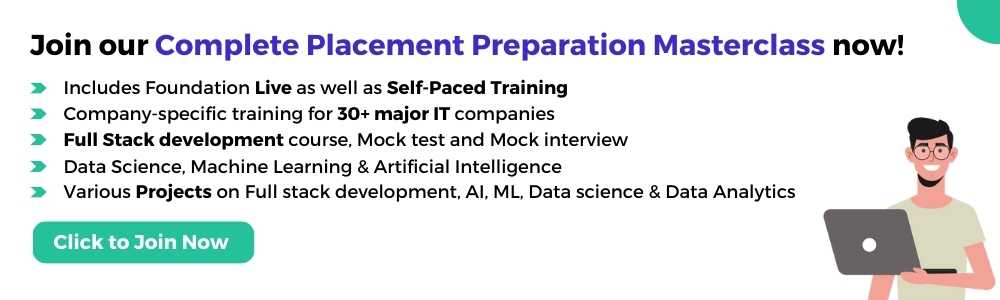
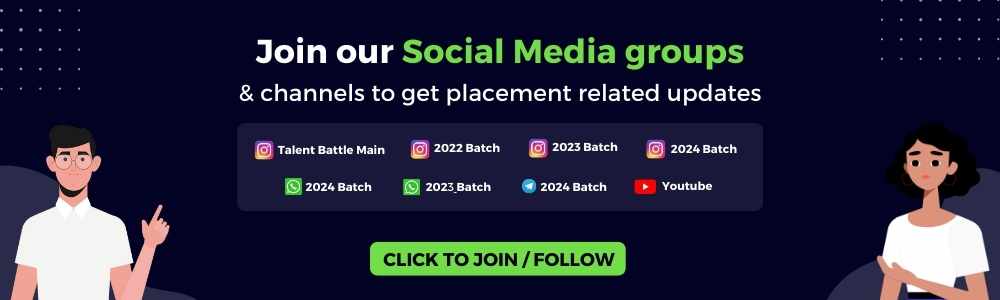