Table of Contents (Click any Topic to view first)
- Java Data Types
- Primitive Data Types
- Non-Primitive Data Types (Reference Types)
- Java - Type Casting
- Widening Type Casting
- Narrowing Type Casting
Understanding data types is fundamental to programming in Java. Data types specify the size and type of values that can be stored in variables. Java is a strongly typed language, which means each variable must be declared with a specific data type. This blog will cover the different data types available in Java, their uses, and how to declare and initialize them.
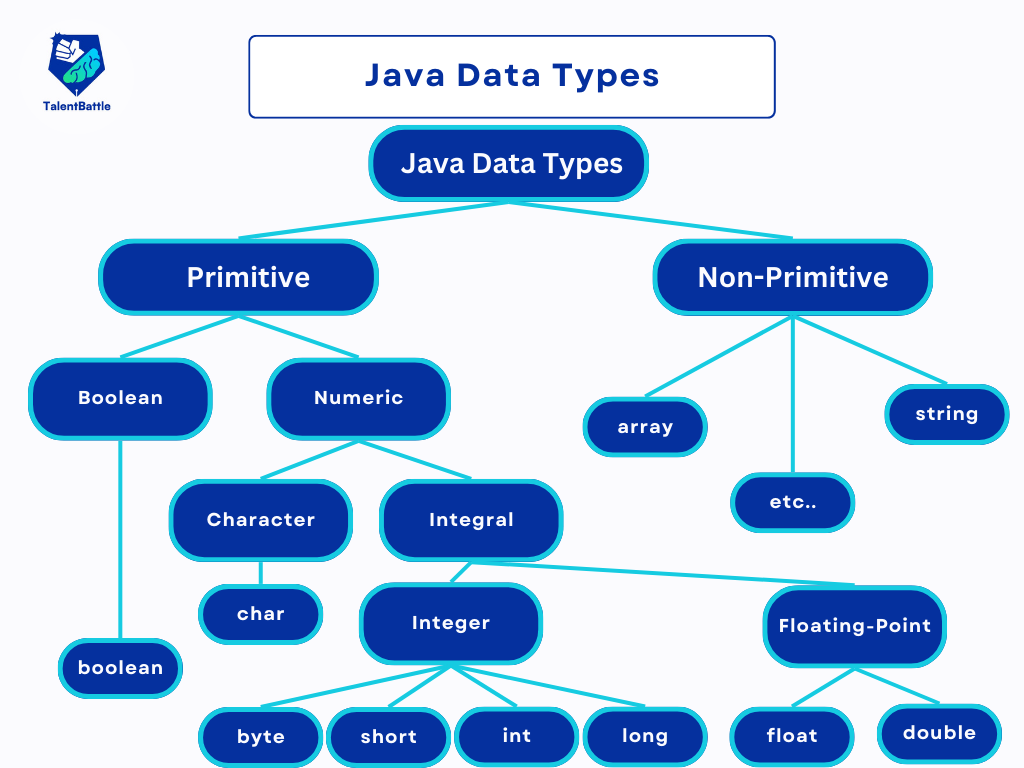
Java provides eight primitive data types which are the building blocks of data manipulation.
- byte
- Size: 8-bit
- Range: -128 to 127
- Use: Saving memory in large arrays where the memory savings are important.
- Example:
byte b = 100;
- short
- Size: 16-bit
- Range: -32,768 to 32,767
- Use: Similar to byte but can store larger numbers.
- Example:
short s = 10000;
- int
- Size: 32-bit
- Range: -2^31 to 2^31-1
- Use: Default data type for integer values.
- Example:
int i = 100000;
- long
- Size: 64-bit
- Range: -2^63 to 2^63-1
- Use: When a wider range than int is needed.
- Example:
long l = 100000L;
- float
- Size: 32-bit IEEE 754 floating-point
- Range: Approximately ±3.40282347E+38F (6-7 significant decimal digits)
- Use: For fractional numbers, when precision up to 6-7 decimal digits is sufficient.
- Example:
float f = 234.5f;
- double
- Size: 64-bit IEEE 754 floating-point
- Range: Approximately ±1.79769313486231570E+308 (15 significant decimal digits)
- Use: For decimal values, usually the default choice for decimal numbers.
- Example:
double d = 123.4;
- boolean
- Size: 1-bit
- Range: Only two possible values:
true
and false
- Use: For simple flags that track true/false conditions.
- Example:
boolean flag = true;
- char
- Size: 16-bit Unicode character
- Range: '\u0000' (or 0) to '\uffff' (or 65,535 inclusive)
- Use: To store characters.
- Example:
char letter = 'A';
Non-primitive data types are also known as reference types because they refer to objects. They are created by the programmer and can be used to access objects. Non-primitive types include classes, interfaces, and arrays.
- Strings
- Definition: A sequence of characters.
- Example:
String message = "Hello, World!";
- Arrays
- Definition: A collection of variables of the same type.
- Example:
int[] numbers = {1, 2, 3, 4, 5};
- Classes
- Definition: A blueprint for creating objects (a particular data structure).
- Example:
public class MyClass {
int x = 5;
}
- Interfaces
- Definition: A reference type, similar to a class, that can contain only constants, method signatures, default methods, static methods, and nested types.
- Example:
interface Animal {
void eat();
void travel();
}
Type Casting
Type casting is when you assign a value of one primitive data type to another type. In Java, there are two types of casting:
- Widening Casting (Implicit)
- Converting a smaller type to a larger type size.
- Example:
int myInt = 9;
double myDouble = myInt; // Automatic casting: int to double
- Narrowing Casting (Explicit)
- Converting a larger type to a smaller size type.
- Example:
double myDouble = 9.78;
int myInt = (int) myDouble; // Manual casting: double to int
Type casting, also known as type conversion, is a method used by either the compiler or the programmer to convert one data type into another. This process is essential when converting types like int
to double
, double
to int
, and short
to int
. Java supports two primary types of casting:
- Widening Type Casting
- Narrowing Type Casting
Widening type casting, also known as implicit type casting, involves converting a smaller data type to a larger data type. This conversion is handled automatically by the compiler and does not require explicit intervention from the programmer.
Widening Type Casting Hierarchy:
byte ->
short ->
char ->
int ->
long ->
float ->
double
The compiler automatically performs this type conversion at compile time, ensuring type safety from smaller to larger data types.
Example 1: Widening Type Casting Error
In the example below, we attempt to store the sum of an int
and a double
into an int
variable, which causes a compilation error.
package com.example;
public class Tester {
public static void main(String[] args) {
int num1 = 5004;
double num2 = 2.5;
int sum = num1 + num2;
// Error: Type mismatch
System.out.println(
"The sum of " + num1 +
" and " + num2 +
" is " + sum);
}
}
Output:
Exception in thread
"main" java.lang.
Error: Unresolved compilation problem:
Type mismatch: cannot convert
from double to
int
Example 2: Correct Widening Type Casting
In this example, the sum of an int
and a double
is stored in a double
variable, allowing the operation to complete successfully.
package com.example;
public class Tester {
public static void main(String[] args) {
int num1 = 5004;
double num2 = 2.5;
double sum = num1 + num2; // No error
System.out.println("The sum of " + num1 + " and " + num2 + " is " + sum);
}
}
Output:
The
sum of
5004 and 2.5 is 5006.5
Narrowing type casting, also known as explicit type casting, is performed manually by the programmer. This type of casting converts a larger data type into a smaller data type and requires the use of a cast operator.
Syntax:
double doubleNum = (double) num;
The above syntax converts the variable num
into a double
.
Example: Narrowing Type Casting
In this example, we first convert an int
to a double
, and then back to an int
, demonstrating explicit type conversion.
package com.example;
public class Tester {
public static void main(String[] args) {
int num = 5004;
double doubleNum = (double) num; // Explicit casting from int to double
System.out.println("The value of " + num + " after converting to double is " + doubleNum);
int convertedInt = (int) doubleNum; // Explicit casting from double to int
System.out.println("The value of " + doubleNum + " after converting back to int is " + convertedInt);
}
}
Output:
The value of 5004 after converting to double is 5004.0
The value of 5004.0 after converting back to int is 5004
Conclusion
Understanding type casting in Java is crucial for managing data type conversions effectively. Widening type casting is handled automatically by the compiler, ensuring safe conversions from smaller to larger data types. In contrast, narrowing type casting requires explicit intervention from the programmer to convert larger data types to smaller ones. Mastering these concepts helps in writing efficient and error-free Java code.
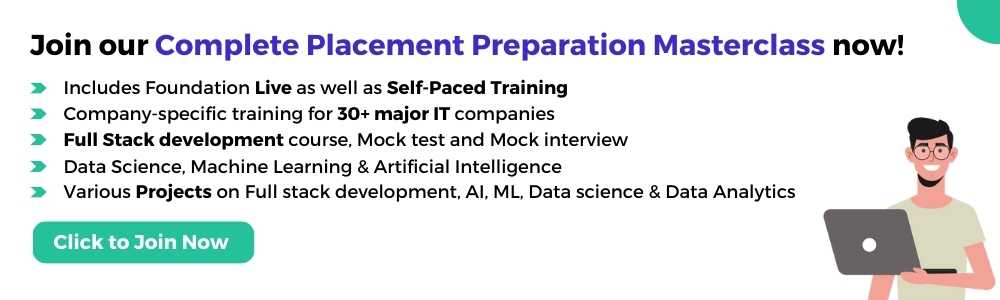
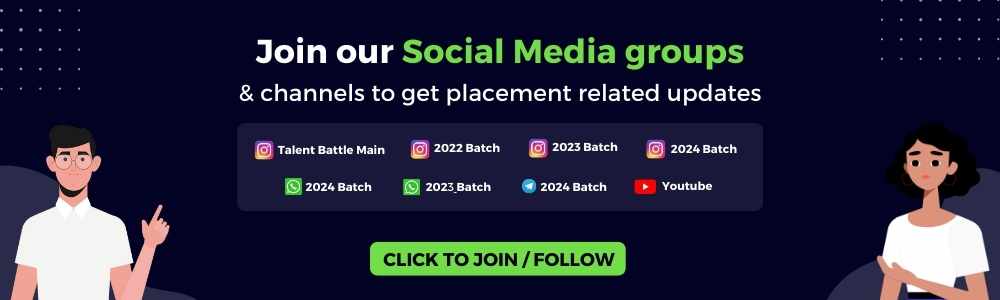