Table of Contents (Click any Topic to view first)
- Syntax of the do-while Loop
- Example 1: Simple do-while Loop
- Example 2: Using do-while Loop for User Input
- Example 3: do-while Loop with Conditional Exit
Understanding the Do While-Loop in Java
In Java programming, the do-while
loop is a variation of the while
loop that ensures the execution of its block of code at least once, regardless of the loop condition. It's useful when you want to execute a block of code and then check the condition for further iterations. Let's explore the do-while
loop in Java with detailed explanations and examples.
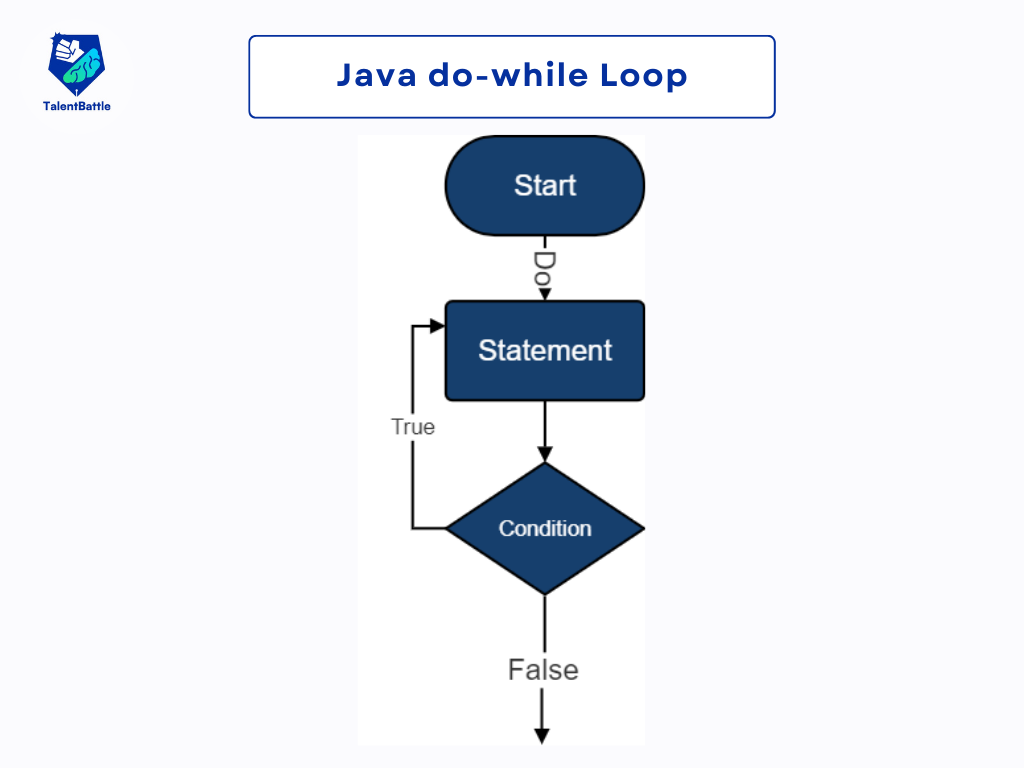
do {
// Code to be executed
} while (condition);
The do-while
loop first executes the block of code inside it, and then evaluates the loop condition. If the condition is true
, the loop continues to execute. The loop terminates when the condition becomes false
.
public class SimpleDoWhileLoop {
public static void main(String[] args) {
int i = 1;
do {
System.out.println("Iteration " + i);
i++;
} while (i <= 5);
}
}
In this example, the do-while
loop iterates from 1 to 5, printing each iteration number. Since the condition (i <= 5
) is evaluated after the first execution, the loop executes at least once even if the condition is initially false
.
import java.util.Scanner;
public class DoWhileLoopUserInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String userInput;
do {
System.out.print("Enter 'exit' to quit: ");
userInput = scanner.nextLine();
System.out.println("You entered: " + userInput);
} while (!userInput.equals("exit"));
scanner.close();
}
}
This example demonstrates the use of a do-while
loop for continuous user input. The loop prompts the user to enter text and prints the input until the user inputs "exit," at which point the loop terminates.
public class ConditionalExitDoWhileLoop {
public static void main(String[] args) {
int i = 1;
do {
System.out.println("Iteration " + i);
if (i == 5) {
break; // Exit the loop when i reaches 5
}
i++;
} while (i <= 10);
}
}
In this example, the loop iterates from 1 to 10, but it exits prematurely when the value of i
becomes 5. The do-while
loop guarantees the execution of the loop body at least once before checking the loop condition.
Conclusion:
The do-while
loop in Java ensures the execution of its block of code at least once, making it suitable for scenarios where you need guaranteed execution before evaluating the loop condition. Whether handling user input, implementing conditional exits, or iterating through a range of values, the do-while
loop offers flexibility and reliability in loop constructs.
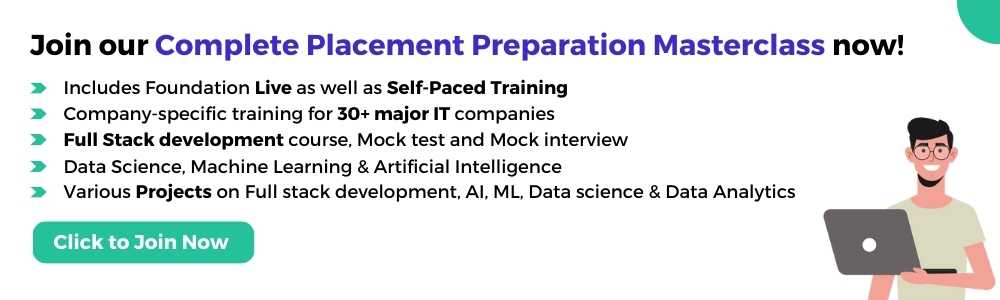
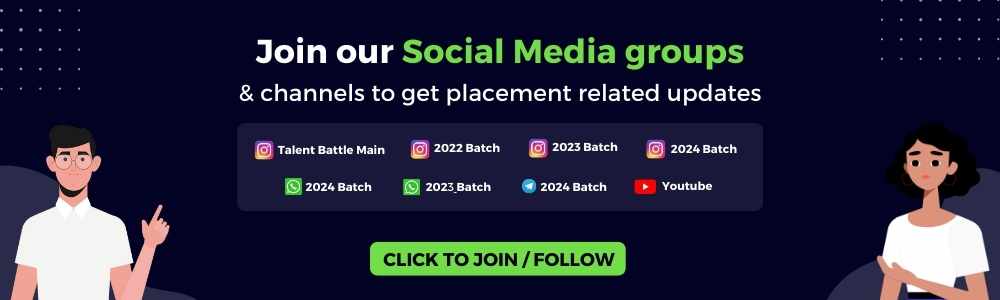