Table of Contents (Click any Topic to view first)
- Key Points of Encapsulation
- Implementation of Encapsulation
- Benefits of Encapsulation
- Real-World Example
Encapsulation in Java is one of the fundamental concepts in object-oriented programming (OOP). It is the mechanism that binds together the data (variables) and the code (methods) that manipulates the data, and keeps both safe from outside interference and misuse. This is typically achieved by making the variables private and providing public getter and setter methods to access and update the values of the private variables.
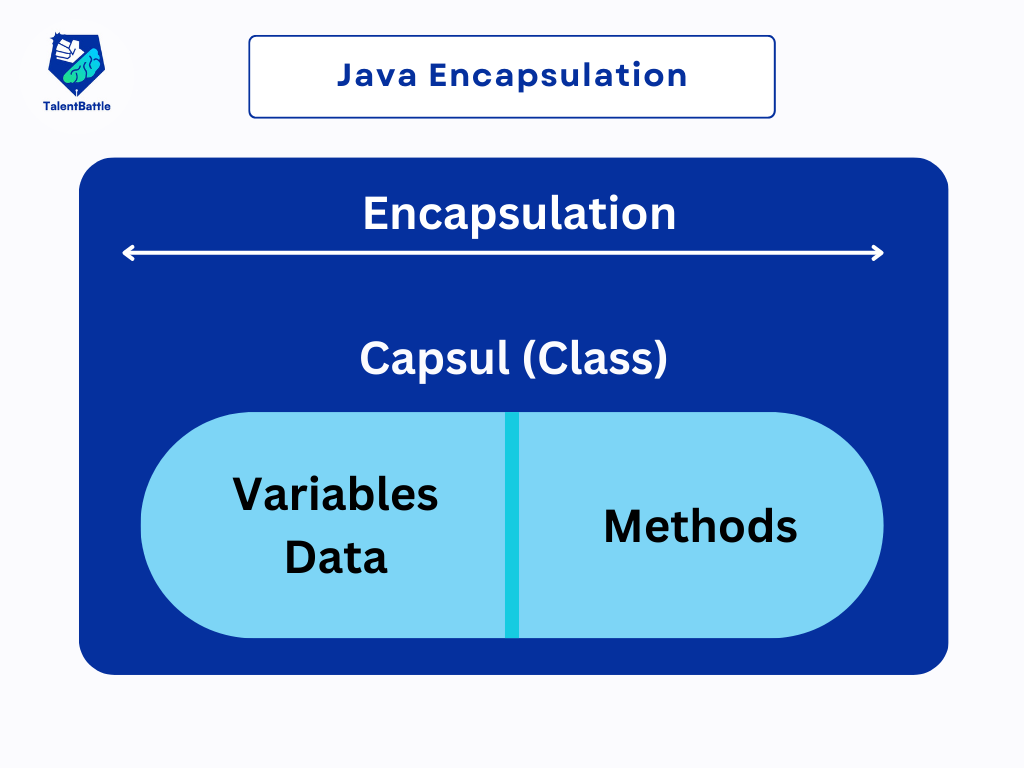
- Data Hiding: The internal state of an object is hidden from the outside. Only the methods of the object can access and modify the state.
- Improves Maintainability: By controlling the access to the internal state, you can change the internal implementation without affecting other parts of the code.
- Enhances Security: Restricts the direct access to some of the object’s components, thus protecting the integrity of the object.
Implementation of Encapsulation
Encapsulation is implemented in Java using:
- Private Variables: The data variables are declared as private to restrict access.
- Public Getter and Setter Methods: Public methods are provided to access and update the values of the private variables.
Example
Let's consider a simple example of encapsulation with a Person class:
public class Person {
// Private variables
private String name;
private int age;
// Public getter for name
public String getName() {
return name;
}
// Public setter for name
public void setName(String name) {
this.name = name;
}
// Public getter for age
public int getAge() {
return age;
}
// Public setter for age
public void setAge(int age) {
if (age > 0) {
this.age = age;
} else {
System.out.println("Age cannot be negative or zero");
}
}
public static void main(String[] args) {
Person p = new Person();
p.setName("John Doe");
p.setAge(30);
System.out.println("Name: " + p.getName());
System.out.println("Age: " + p.getAge());
}
}
Explanation
- Private Variables: The variables name and age are private, meaning they cannot be accessed directly from outside the Person class.
- Public Methods: The getName, setName, getAge, and setAge methods are public, providing controlled access to the private variables.
- Validation: The setAge method includes a simple validation to ensure the age is not negative or zero, showcasing how encapsulation can be used to enforce rules on the data.
- Controlled Access: By using getter and setter methods, you can control how the important data variables are accessed and modified.
- Increased Flexibility: You can change the internal implementation without affecting other parts of the code that use the class.
- Reusability: Encapsulated code is easier to reuse and modify.
- Maintainability: Encapsulation makes it easier to manage and maintain the code since the data and the methods that manipulate the data are kept together.
Real-World Example
Consider a BankAccount class that encapsulates account balance and provides methods to deposit and withdraw money:
public class BankAccount {
// Private variable to store account balance
private double balance;
// Constructor to initialize balance
public BankAccount(double initialBalance) {
if (initialBalance > 0) {
this.balance = initialBalance;
} else {
this.balance = 0;
}
}
// Public method to deposit money
public void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
// Public method to withdraw money
public void withdraw(double amount) {
if (amount > 0 && amount <= balance) {
balance -= amount;
} else {
System.out.println("Invalid withdrawal amount or insufficient funds");
}
}
// Public method to check balance
public double getBalance() {
return balance;
}
public static void main(String[] args) {
BankAccount account = new BankAccount(1000);
account.deposit(500);
account.withdraw(200);
System.out.println("Current balance: " + account.getBalance());
}
}
Explanation
- Private Variable: The balance variable is private to restrict direct access.
- Public Methods: The deposit, withdraw, and getBalance methods provide controlled access to modify and retrieve the balance.
- Constructor Validation: The constructor ensures the initial balance is positive.
Conclusion
Encapsulation in Java is a powerful mechanism that enhances the security, maintainability, and flexibility of your code. By using private variables and providing public getter and setter methods, you can control how the data is accessed and modified, ensuring the integrity of the data and making the code easier to manage and maintain.
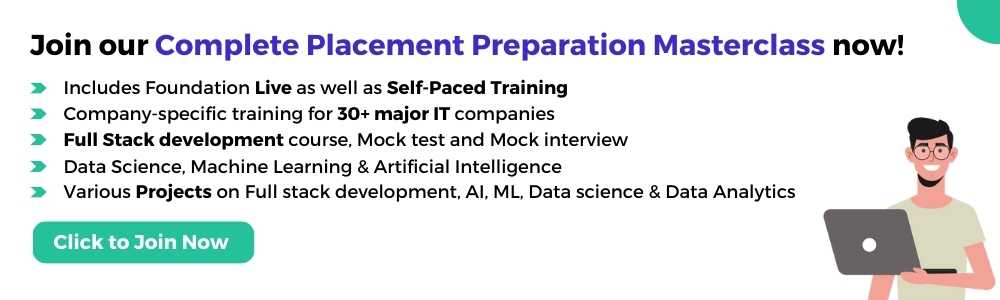
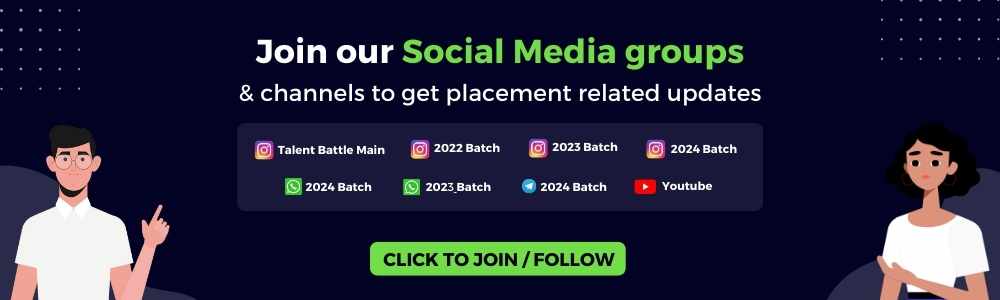