Table of Contents (Click any Topic to view first)
- Try-Catch Blocks
- Checked vs. Unchecked Exceptions
- Custom Exceptions
- Key Concepts
Exception handling in Java is a robust mechanism for handling runtime errors so that the normal flow of the application can be maintained. Java provides a way to handle exceptions using try
, catch
, finally
, throw
, and throws
keywords.
The try-catch
block is used to handle exceptions. The code that might throw an exception is placed inside the try
block. The catch
block is used to handle the exception.
Syntax:
try {
// code that may throw an exception
} catch (ExceptionType e) {
// code to handle the exception
}
Example:
public class TryCatchExample {
public static void main(String[] args) {
try {
int data = 50 / 0; // may throw an exception
} catch (ArithmeticException e) {
System.out.println("ArithmeticException: Division by zero!");
}
System.out.println("Rest of the code...");
}
}
Checked Exceptions:
Checked exceptions are checked at compile-time. These exceptions must be either caught or declared in the method using the throws
keyword. Common checked exceptions include IOException
, SQLException
, etc.
Example:
import java.io.*;
public class CheckedExceptionExample {
public static void main(String[] args) {
try {
FileInputStream file = new FileInputStream("test.txt");
} catch (FileNotFoundException e) {
System.out.println("FileNotFoundException: File not found");
}
}
}
Unchecked Exceptions:
Unchecked exceptions are not checked at compile-time, but they are checked at runtime. They are also known as runtime exceptions. Common unchecked exceptions include ArithmeticException
, NullPointerException
, ArrayIndexOutOfBoundsException
, etc.
Example:
public class UncheckedExceptionExample {
public static void main(String[] args) {
try {
int data = 50 / 0; // may throw an exception
} catch (ArithmeticException e) {
System.out.println("ArithmeticException: Division by zero!");
}
System.out.println("Rest of the code...");
}
}
Java allows users to create their own exceptions. Custom exceptions are useful when we want to handle specific error conditions.
Steps to Create a Custom Exception:
- Create a class that extends
Exception
(for checked exceptions) or RuntimeException
(for unchecked exceptions).
- Provide one or more constructors in your custom exception class.
Example:
// Creating a custom checked exception
class InvalidAgeException extends Exception {
public InvalidAgeException(String message) {
super(message);
}
}
// Using the custom exception
public class CustomExceptionExample {
static void validate(int age) throws InvalidAgeException {
if (age < 18) {
throw new InvalidAgeException("Age is not valid for voting");
} else {
System.out.println("Welcome to vote");
}
}
public static void main(String[] args) {
try {
validate(16);
} catch (InvalidAgeException e) {
System.out.println("Caught the exception: " + e.getMessage());
}
System.out.println("Rest of the code...");
}
}
finally
Block:
The finally
block is used to execute important code such as closing resources. It always executes, whether an exception is handled or not.
Example:
public class FinallyBlockExample {
public static void main(String[] args) {
try {
int data = 25 / 0;
} catch (ArithmeticException e) {
System.out.println("Exception caught: " + e);
} finally {
System.out.println("Finally block is always executed");
}
}
}
throw
Keyword:
The throw
keyword is used to explicitly throw an exception.
Example:
public class ThrowExample {
static void checkAge(int age) {
if (age < 18) {
throw new ArithmeticException("Not eligible for voting");
} else {
System.out.println("Eligible for voting");
}
}
public static void main(String[] args) {
checkAge(16);
System.out.println("Rest of the code...");
}
}
throws
Keyword:
The throws
keyword is used in the method signature to declare that a method can throw an exception.
Example:
import java.io.*;
public class ThrowsExample {
void method() throws IOException {
throw new IOException("Device error");
}
public static void main(String[] args) {
try {
ThrowsExample obj = new ThrowsExample();
obj.method();
} catch (IOException e) {
System.out.println("Exception handled: " + e);
}
System.out.println("Rest of the code...");
}
}
Conclusion
Exception handling in Java is a powerful mechanism to handle runtime errors and maintain the normal flow of the application. By using try
, catch
, finally
, throw
, and throws
, you can handle both checked and unchecked exceptions effectively. Custom exceptions allow you to handle specific error conditions in your application, making your code more robust and maintainable.
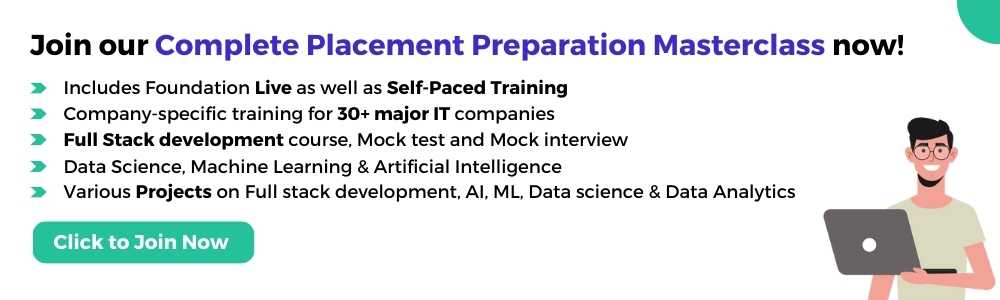
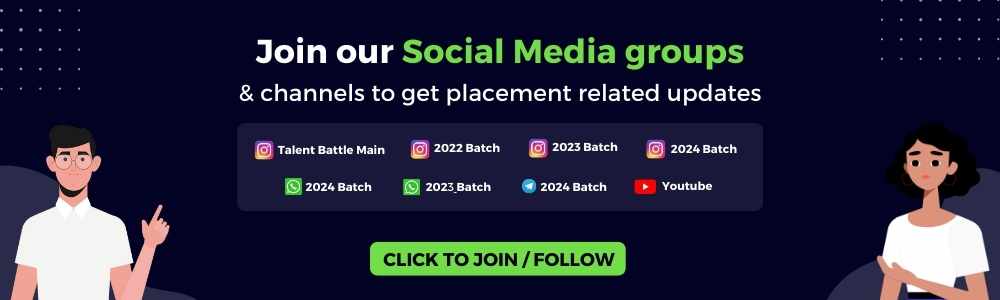