Table of Contents (Click any Topic to view first)
- Basic Syntax of the For-Loop
- Enhanced For-Loop (For-Each Loop)
- Common Use Cases for the For-Loop
Understanding the For-Loop in Java
The for-loop is a fundamental control structure in Java, enabling developers to execute a block of code repeatedly with a counter that controls the number of iterations. It is particularly useful when you know in advance how many times you need to execute a statement or a block of statements. In this blog, we'll dive deep into the for-loop, exploring its syntax, usage, and some practical examples.
Basic Syntax of the For-Loop
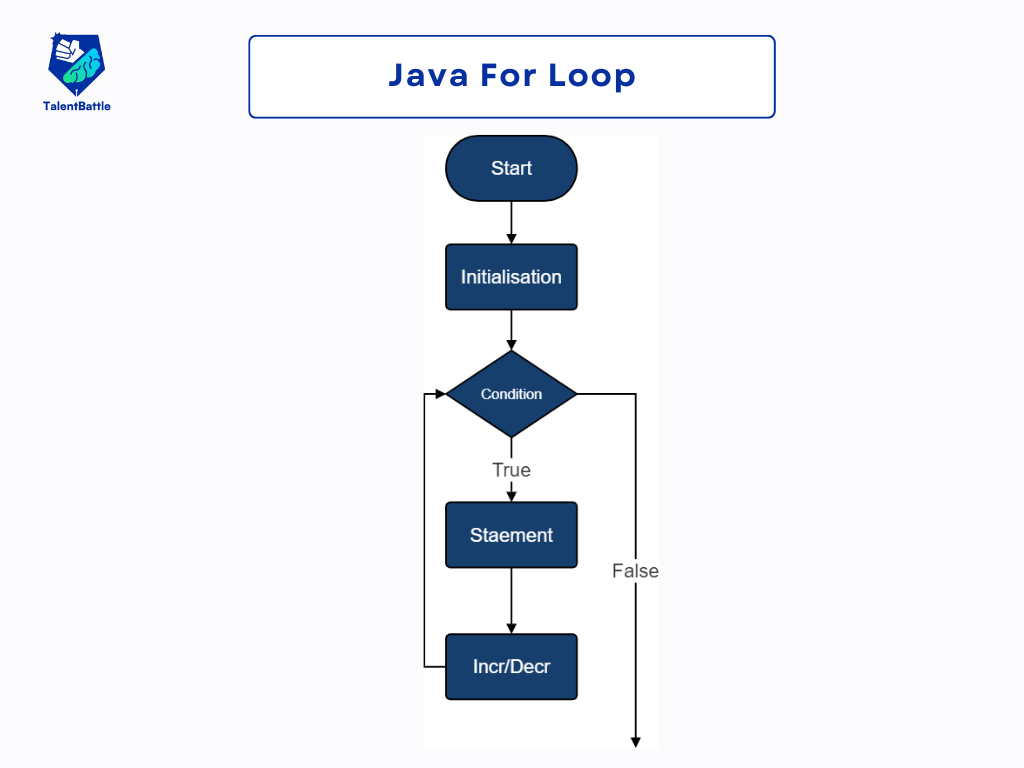
The basic syntax of a for-loop in Java looks like this:
for (initialization; condition; update) {
// code to be executed
}
Let's break down each part of the for-loop:
-
Initialization: This is where you initialize the loop control variable. It is executed only once, at the beginning of the loop.
-
Condition: This is a boolean expression that is checked before each iteration. If the condition evaluates to true, the loop's body is executed. If it evaluates to false, the loop terminates.
-
Update: This is executed after each iteration of the loop. It is typically used to update the loop control variable.
Here is a simple example to illustrate the basic for-loop:
public class ForLoopExample {
public static void main(String[] args) {
for (int i = 0; i < 5; i++) {
System.out.println("Iteration: " + i);
}
}
}
In this example:
-
The loop starts with i
initialized to 0.
-
The condition i < 5
is checked before each iteration.
-
After each iteration, i
is incremented by 1.
The output will be:
Iteration: 0
Iteration: 1
Iteration: 2
Iteration: 3
Iteration: 4
Java also provides an enhanced for-loop, commonly known as the for-each loop, which is used to iterate over arrays or collections. The syntax is simpler and more readable:
for (type variable : array) {
// code to be executed
}
Here's an example using an array of integers:
public class EnhancedForLoopExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
System.out.println("Number: " + number);
}
}
}
The output will be:
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
-
Iterating over Arrays: The for-loop is commonly used to iterate over arrays and perform operations on each element.
int[] numbers = {10, 20, 30, 40, 50};
for (int i = 0; i < numbers.length; i++) {
numbers[i] = numbers[i] * 2;
}
-
Generating Sequences: The for-loop can be used to generate sequences of numbers.
for (int i = 1; i <= 10; i++) {
System.out.println("Number: " + i);
}
-
Nested For-Loops: For-loops can be nested to handle more complex iterations, such as working with multi-dimensional arrays.
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
System.out.print(matrix[i][j] + " ");
}
System.out.println();
}
The output will be:
1 2 3
4 5 6
7 8 9
Tips for Using For-Loops
// This will create an infinite loop
for (int i = 0; i >= 0; i++) {
System.out.println("This will run forever!");
}
for (int index = 0; index < array.length; index++) {
// Do something with array[index]
}
Conclusion
The for-loop is a powerful and versatile tool in Java programming. By understanding its syntax and various use cases, you can write more efficient and readable code. Whether you are iterating over arrays, generating sequences, or handling complex data structures, the for-loop is an indispensable part of your Java toolkit. Happy coding!
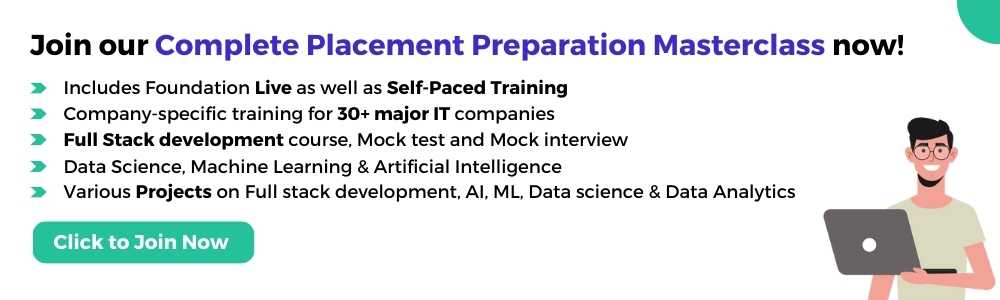
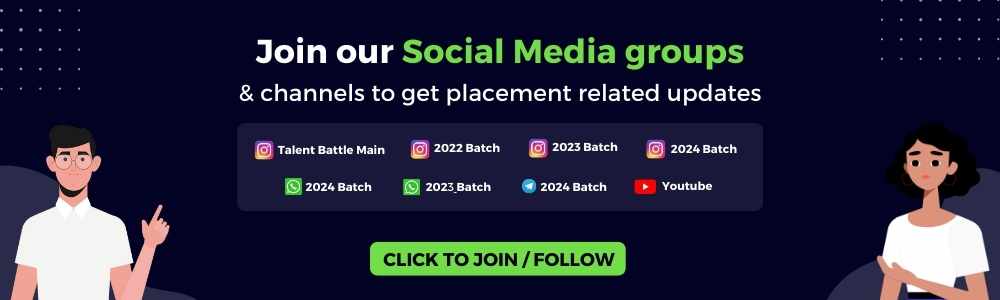