Table of Contents (Click any Topic to view first)
- Writing the Program
- Breaking Down the Code
- Running the Program
- Conclusion
Java Program to Print "Hello World"
Welcome to the world of Java programming! If you're just starting out with Java, one of the first things you'll learn is how to write a simple program that prints "Hello, World!" to the console. This classic introductory program is a great way to get familiar with the basics of Java syntax and structure. Let's dive in and write your first Java program together.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
public class HelloWorld {
: This line declares a class named HelloWorld. In Java, every program must have at least one class, and the filename must match the class name.
public static void main(String[] args) {:
This line defines the main method, which is the entry point for any Java program. The public keyword indicates that the method can be accessed from anywhere, static means the method belongs to the class itself rather than to any specific object of the class, void specifies that the method doesn't return any value, and String[] args is an array of strings that can be passed as arguments when the program is executed.
System.out.println("Hello, World!");
: This line uses the System.out.println() method to print the string "Hello, World!" to the console. System.out refers to the standard output stream, and println() is a method used to print a line of text.
To run this program, follow these steps:
- Open a text editor and copy the code above into a new file named HelloWorld.java.
- Open a command prompt or terminal window.
- Navigate to the directory where you saved the HelloWorld.java file.
- Compile the program by typing javac HelloWorld.java and pressing Enter.
- If the compilation is successful, run the program by typing java HelloWorld and pressing Enter.
- You should see the output Hello, World! printed to the console.
Congratulations! You've just written and executed your first Java program. This simple example demonstrates the basic structure of a Java program and introduces you to some fundamental concepts of the Java language. From here, you can continue to explore and learn more about Java programming, including object-oriented principles, data structures, algorithms, and much more. Happy coding!
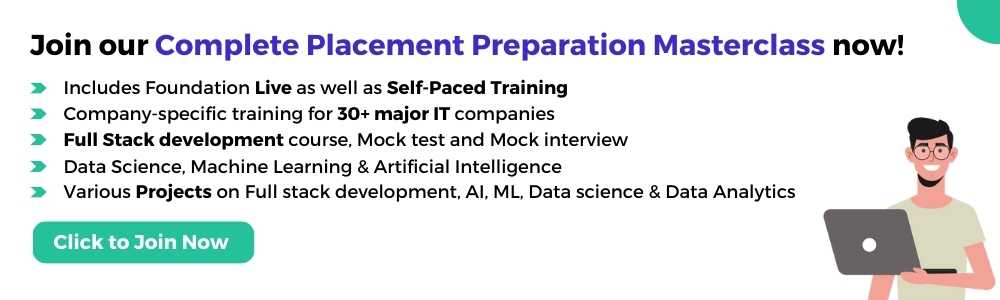
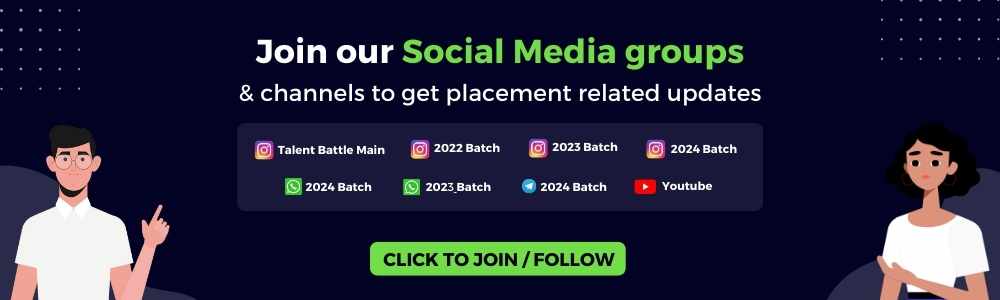