Table of Contents (Click any Topic to view first)
- If-else Statement Syntax:
- Example 1: Simple If-else Statement
- Example 2: Nested If-else Statement
- Example 3: Chained If-else Statement (if-else-if)
- Example 4: If-else Statement with User Input
In Java programming, decision-making statements allow you to control the flow of your program based on specific conditions. One of the fundamental decision-making statements in Java is the if-else statement. This statement evaluates a condition and executes different blocks of code depending on whether the condition is true or false. Let's explore the if-else statement in detail with examples.
if (condition) {
// Code block executed if condition is true
} else {
// Code block executed if condition is false
}
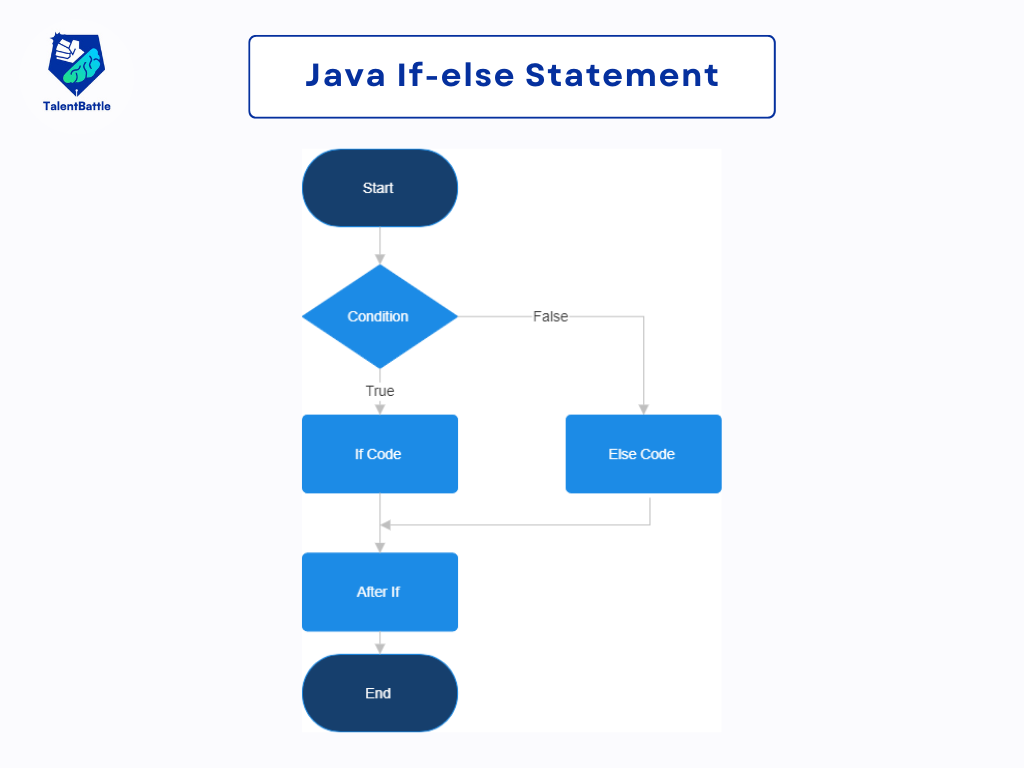
public class IfElseExample {
public static void main(String[] args) {
int x = 10;
if (x % 2 == 0) {
System.out.println("x is even");
} else {
System.out.println("x is odd");
}
}
}
In this example, if the value of x
is divisible by 2 (i.e., it's even), the program prints "x is even." Otherwise, it prints "x is odd."
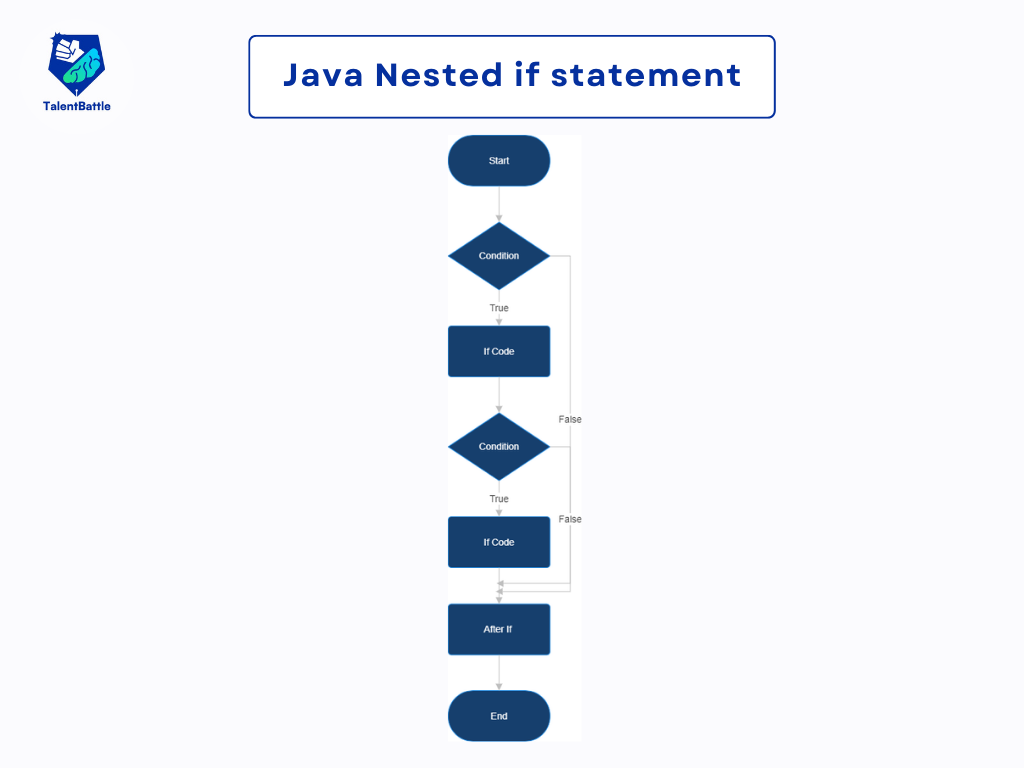
public class NestedIfElseExample {
public static void main(String[] args) {
int age = 25;
if (age >= 18) {
if (age <= 30) {
System.out.println("Age is between 18 and 30");
} else {
System.out.println("Age is greater than 30");
}
} else {
System.out.println("Age is less than 18");
}
}
}
This example demonstrates a nested if-else statement. If the age is greater than or equal to 18, it checks if the age is also less than or equal to 30. Based on these conditions, it prints different messages.
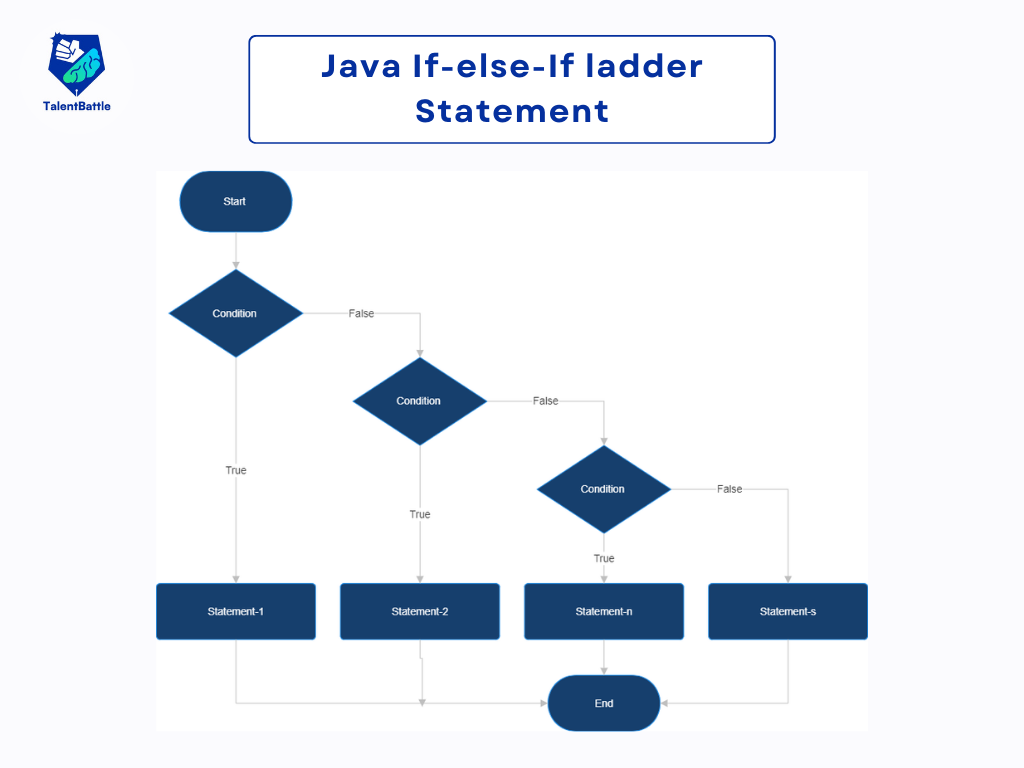
public class ElseIfExample {
public static void main(String[] args) {
int marks = 85;
if (marks >= 90) {
System.out.println("Grade: A");
} else if (marks >= 80) {
System.out.println("Grade: B");
} else if (marks >= 70) {
System.out.println("Grade: C");
} else {
System.out.println("Grade: D");
}
}
}
In this example, the program evaluates the marks and assigns a grade based on the score. It uses chained if-else-if statements to check multiple conditions in sequence.
import java.util.Scanner;
public class UserInputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your age: ");
int age = scanner.nextInt();
if (age >= 18) {
System.out.println("You are eligible to vote");
} else {
System.out.println("You are not eligible to vote");
}
scanner.close();
}
}
This example demonstrates how to use the if-else statement with user input. It prompts the user to enter their age and then determines whether they are eligible to vote based on the entered age.
Conclusion:
The if-else statement is a powerful tool in Java for making decisions in your programs. Whether it's simple conditions, nested scenarios, or chained evaluations, the if-else statement provides flexibility and control over the flow of your code. Understanding its syntax and usage is essential for writing efficient and effective Java programs.
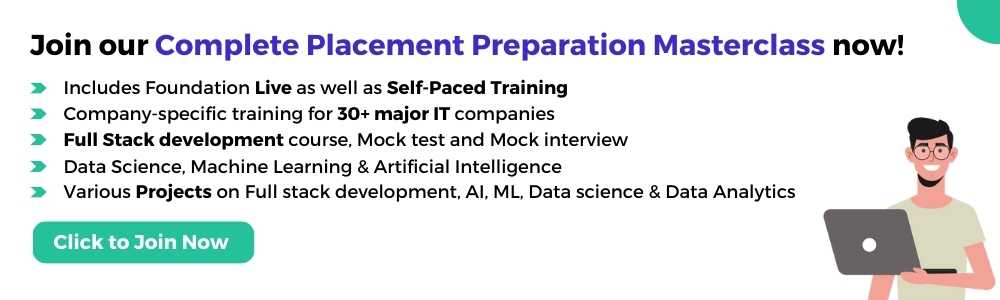
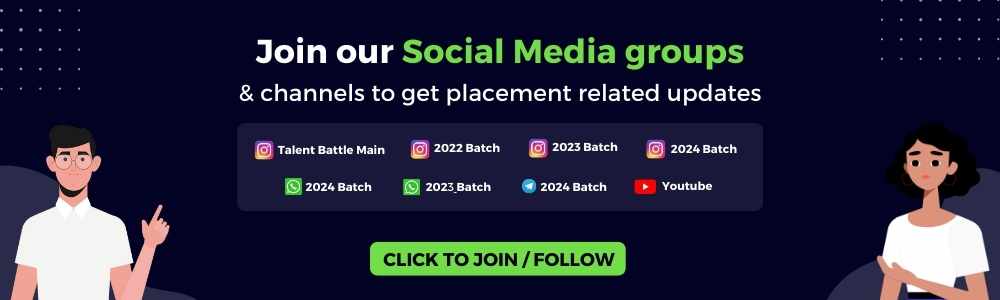