Table of Contents (Click any Topic to view first)
- What is Inheritance?
- Why Use Inheritance?
- Key Terms in Inheritance
- Syntax of Inheritance
- Example of Inheritance in Java
- Types of Inheritance in Java
- Why Multiple Inheritance is Not Supported for Classes
- Hybrid Inheritance in Java
Understanding the Inheritance in Java
Inheritance is a fundamental concept in Java, allowing one class to acquire the properties and behaviors of another class. This mechanism is central to Object-Oriented Programming (OOP) and enables code reusability and polymorphism.
Inheritance in Java is a mechanism where a new class, known as a child or subclass, derives attributes and methods from an existing class, known as a parent or superclass. This relationship is often described as an "IS-A" relationship, indicating that the child class is a type of the parent class.
- Method Overriding: Enables runtime polymorphism.
- Code Reusability: Allows the reuse of existing code, reducing redundancy.
- Class: A blueprint for creating objects, defining properties and behaviors.
- Sub Class/Child Class: Inherits from another class, also known as derived or extended class.
- Super Class/Parent Class: The class being inherited from, also known as the base class.
- Reusability: Using fields and methods of an existing class in a new class.
class Subclass-name extends Superclass-name {
// methods and fields
}
The extends
keyword indicates that the new class is derived from an existing class.
In the example below, Programmer
is a subclass of Employee
.
class Employee {
float salary = 40000;
}
class Programmer extends Employee {
int bonus = 10000;
public static void main(String args[]) {
Programmer p = new Programmer();
System.out.println("Programmer salary is: " + p.salary);
System.out.println("Bonus of Programmer is: " + p.bonus);
}
}
Output:
Programmer salary is: 40000.0
Bonus of Programmer is: 10000
Java supports three types of inheritance based on class relationships: single, multilevel, and hierarchical. Multiple inheritance is supported through interfaces.
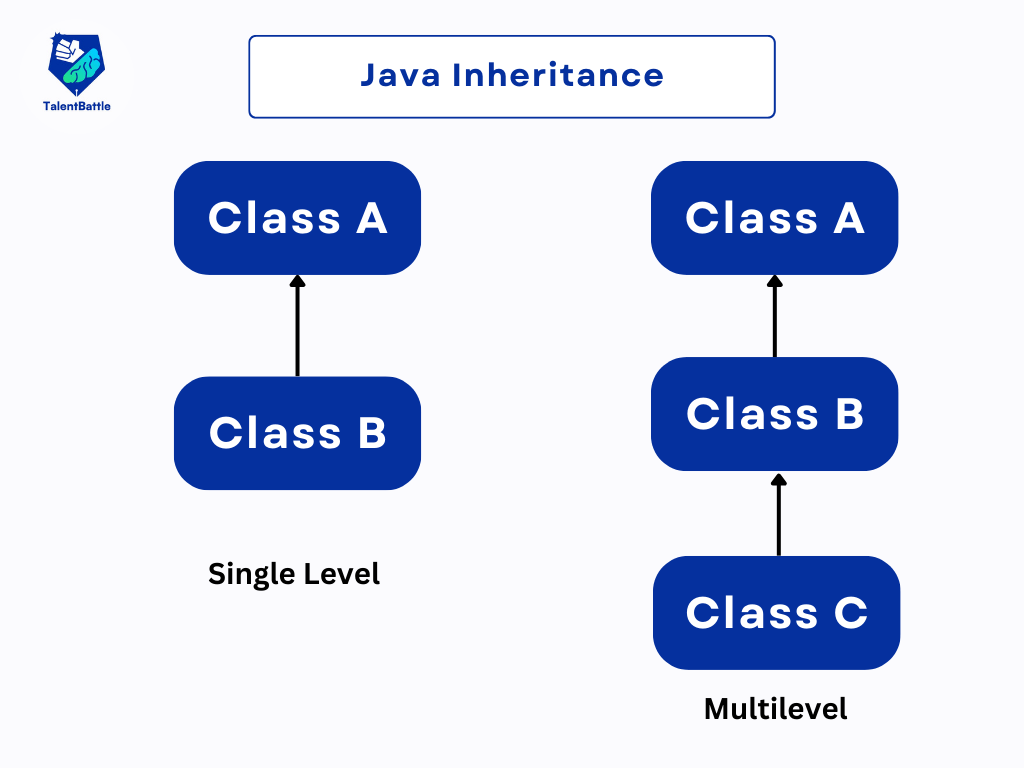
1. Single Inheritance
A class inherits from one superclass.
class Animal {
void eat() { System.out.println("eating..."); }
}
class Dog extends Animal {
void bark() { System.out.println("barking..."); }
}
class TestInheritance {
public static void main(String args[]) {
Dog d = new Dog();
d.bark();
d.eat();
}
}
Output:
barking...
eating...
2. Multilevel Inheritance
A class is derived from a class which is also derived from another class.
class Animal {
void eat() { System.out.println("eating..."); }
}
class Dog extends Animal {
void bark() { System.out.println("barking..."); }
}
class BabyDog extends Dog {
void weep() { System.out.println("weeping..."); }
}
class TestInheritance2 {
public static void main(String args[]) {
BabyDog d = new BabyDog();
d.weep();
d.bark();
d.eat();
}
}
Output:
weeping...
barking...
eating...
.png)
3. Hierarchical Inheritance
Multiple classes inherit from a single superclass.
class Animal {
void eat() { System.out.println("eating..."); }
}
class Dog extends Animal {
void bark() { System.out.println("barking..."); }
}
class Cat extends Animal {
void meow() { System.out.println("meowing..."); }
}
class TestInheritance3 {
public static void main(String args[]) {
Cat c = new Cat();
c.meow();
c.eat();
// c.bark(); // Compile Time Error
}
}
Output:
meowing...
eating...
Java does not support multiple inheritance through classes to avoid complexity and ambiguity. If a class inherits from multiple classes that have methods with the same signature, it creates a conflict about which method to execute.
Consider the scenario with classes A
, B
, and C
. If C
inherits both A
and B
and both have a method msg()
, there would be ambiguity in calling msg()
from C
.
class A {
void msg() { System.out.println("Hello"); }
}
class B {
void msg() { System.out.println("Welcome"); }
}
class C extends A, B { // Suppose if it were possible
public static void main(String args[]) {
C obj = new C();
obj.msg(); // Which msg() method would be invoked?
}
}
Output:
Compile Time Error: Multiple inheritance is not supported in Java
To avoid such conflicts and keep the language simple, Java allows multiple inheritance only through interfaces, ensuring compile-time safety and reducing potential runtime errors.
.png)
Hybrid inheritance is a combination of two or more types of inheritance (single, multilevel, hierarchical). Although Java does not support multiple inheritance with classes to avoid complexity and ambiguity, hybrid inheritance can be achieved using interfaces.
interface Animal {
void eat();
}
interface Pet {
void play();
}
class Dog implements Animal, Pet {
public void eat() {
System.out.println("eating...");
}
public void play() {
System.out.println("playing...");
}
}
class TestHybridInheritance {
public static void main(String args[]) {
Dog d = new Dog();
d.eat();
d.play();
}
}
Conclusion
Inheritance is a cornerstone of Object-Oriented Programming in Java, enabling code reusability, scalability, and polymorphism. By using inheritance, developers can create a hierarchy of classes that share common behavior while allowing for specific functionality in subclasses. Despite the restriction on multiple inheritance with classes to maintain simplicity, Java provides robust support for inheritance through interfaces, thus ensuring flexibility and reducing potential errors. Understanding and effectively implementing inheritance is crucial for designing efficient and maintainable Java applications.
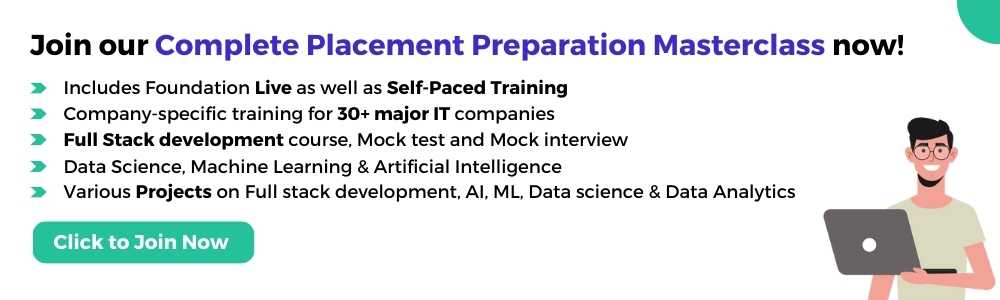
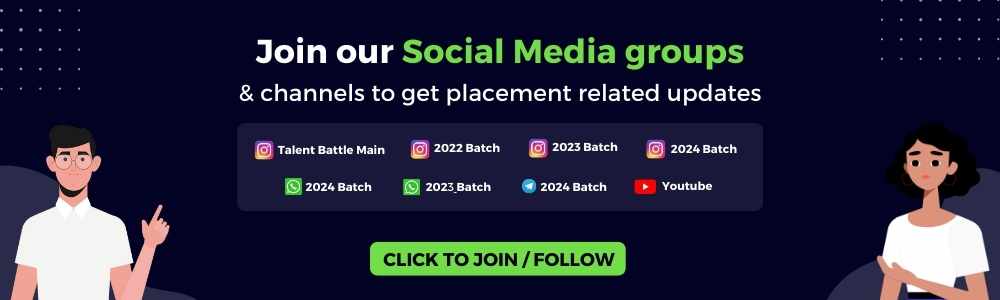