Table of Contents (Click any Topic to view first)
- File Handling in Java
- Reading from and Writing to Files
- Serialization and Deserialization
Java I/O (Input and Output) is a fundamental concept that deals with the reading and writing of data (text, binary, or objects). The java.io
package provides classes and interfaces for system input and output through data streams, serialization, and the file system.
File handling in Java involves creating, reading, writing, and deleting files and directories. The java.io.File
class provides methods for file handling.
Creating a File
To create a file, you can use the createNewFile()
method of the File
class.
Example:
import java.io.File;
import java.io.IOException;
public class FileCreateExample {
public static void main(String[] args) {
File file = new File("example.txt");
try {
if (file.createNewFile()) {
System.out.println("File created: " + file.getName());
} else {
System.out.println("File already exists.");
}
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
Deleting a File
To delete a file, you can use the delete()
method of the File
class.
Example:
import java.io.File;
public class FileDeleteExample {
public static void main(String[] args) {
File file = new File("example.txt");
if (file.delete()) {
System.out.println("Deleted the file: " + file.getName());
} else {
System.out.println("Failed to delete the file.");
}
}
}
Java provides several classes for reading from and writing to files, including FileReader
, FileWriter
, BufferedReader
, BufferedWriter
, and Scanner
.
Writing to a File
You can use the FileWriter
and BufferedWriter
classes to write data to a file.
Example:
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
public class FileWriteExample {
public static void main(String[] args) {
try (BufferedWriter writer = new BufferedWriter(new FileWriter("example.txt"))) {
writer.write("Hello, world!");
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
Reading from a File
You can use the FileReader
, BufferedReader
, and Scanner
classes to read data from a file.
Example with BufferedReader
:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadExample {
public static void main(String[] args) {
try (BufferedReader reader = new BufferedReader(new FileReader("example.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
Example with Scanner
:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class FileReadExampleScanner {
public static void main(String[] args) {
try (Scanner scanner = new Scanner(new File("example.txt"))) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
System.out.println(line);
}
} catch (FileNotFoundException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
Serialization is the process of converting an object into a byte stream, and deserialization is the reverse process, where the byte stream is converted back into a copy of the object. This is useful for saving objects to files or sending them over a network.
Serialization
To serialize an object, the class must implement the Serializable
interface. You can use ObjectOutputStream
to write the object to a file.
Example:
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.io.Serializable;
class Person implements Serializable {
private static final long serialVersionUID = 1L;
String name;
int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
}
public class SerializationExample {
public static void main(String[] args) {
Person person = new Person("John", 30);
try (FileOutputStream fileOut = new FileOutputStream("person.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut)) {
out.writeObject(person);
System.out.println("Serialized data is saved in person.ser");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Deserialization
To deserialize an object, you can use ObjectInputStream
to read the object from a file.
Example:
import java.io.FileInputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
public class DeserializationExample {
public static void main(String[] args) {
try (FileInputStream fileIn = new FileInputStream("person.ser");
ObjectInputStream in = new ObjectInputStream(fileIn)) {
Person person = (Person) in.readObject();
System.out.println("Deserialized Person...");
System.out.println("Name: " + person.name);
System.out.println("Age: " + person.age);
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
Conclusion
Java I/O is a critical aspect of Java programming, allowing for effective file handling, data reading and writing, and object serialization and deserialization. Mastery of these concepts enables developers to handle data input and output operations efficiently in their applications.
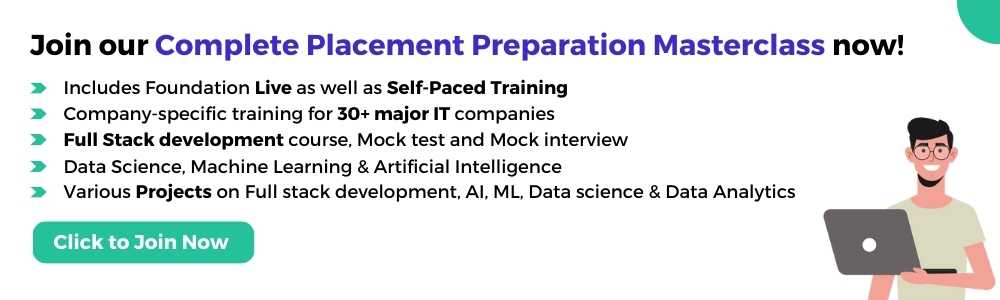
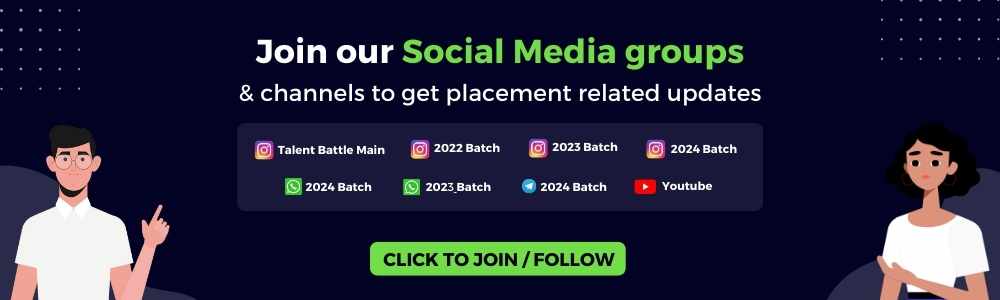