Table of Contents (Click any Topic to view first)
- Definition of Methods
- Types of Methods
- Example: Defining and Invoking a Method in Java
- Key Concepts
- Definition of Constructors
- Types of Constructors
- Example: Implementing Constructors in Java
- Key Concepts of Constructors
Understanding the Methods and Constructors in Java
In Java programming, methods are essential building blocks used to encapsulate reusable code and perform specific tasks. Understanding how to define, invoke, and utilize methods is crucial for developing efficient and organized Java applications. Let's explore methods in Java comprehensively, covering their syntax, types, and usage with illustrative examples.
- Definition: A method in Java is a collection of statements that perform a specific task. It is defined within a class and can be called (invoked) to execute its functionality.
- Syntax:
returnType methodName(parameter1Type parameter1, parameter2Type parameter2, ...) {
// Method body
// Statements
return returnValue; // Optional return statement
}
a. Void Methods:
- Definition: Void methods do not return any value. They perform a task without producing a result.
- Syntax:
void methodName(parameter1Type parameter1, parameter2Type parameter2, ...) {
// Method body
// Statements
}
b. Methods with Return Type:
- Definition: Methods with a return type return a value after performing a task.
- Syntax:
returnType methodName(parameter1Type parameter1, parameter2Type parameter2, ...) {
// Method body
// Statements
return returnValue; // Return statement with a value
}
public class Calculator {
// Method to add two numbers and return the result
public int add(int num1, int num2) {
int sum = num1 + num2;
return sum;
}
// Method to display a message
public void displayMessage(String message) {
System.out.println(message);
}
}
public class Main {
public static void main(String[] args) {
// Creating an object of the Calculator class
Calculator calculator = new Calculator();
// Invoking the add method and storing the result
int result = calculator.add(5, 3);
System.out.println("Result of addition: " + result);
// Invoking the displayMessage method
calculator.displayMessage("Hello, Java!");
}
}
- Method Signature: Consists of the method name and parameter list. It uniquely identifies the method within a class.
- Parameters: Input values passed to the method for processing.
- Return Type: Specifies the type of value returned by the method.
- Return Statement: Terminates the execution of a method and returns a value (if applicable) to the caller.
- Method Overloading: Defining multiple methods with the same name but different parameter lists.
Conclusion:
Methods are essential components of Java programming, allowing developers to encapsulate logic, improve code readability, and promote code reusability. By mastering methods, Java developers can write modular and maintainable code for a wide range of applications.
Constructors in Java:-
Constructors play a pivotal role in object-oriented programming in Java, as they facilitate the initialization of objects. Understanding how constructors work and how to utilize them effectively is essential for Java developers. Let's delve into constructors in Java, covering their definition, types, usage, and best practices.
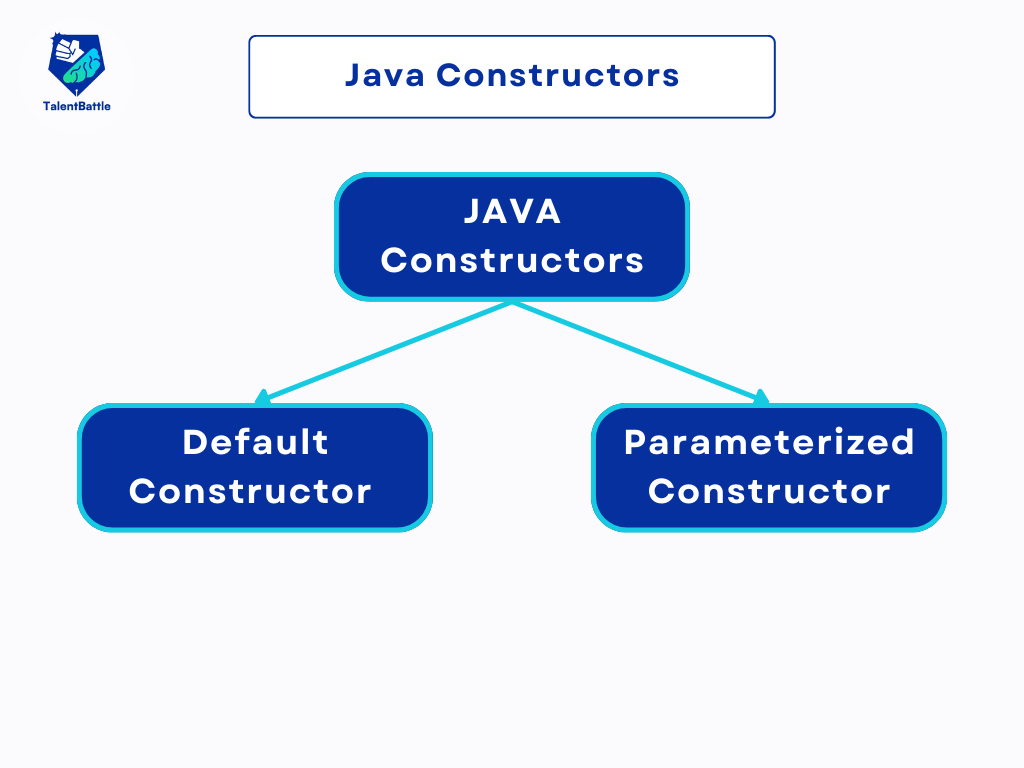
- Definition: A constructor in Java is a special type of method that is invoked when an object of a class is created. It initializes the newly created object and prepares it for use.
- Syntax:
public ClassName(parameter1Type parameter1, parameter2Type parameter2, ...) {
// Constructor body
// Initialization code
}
2. Types of Constructors:
a. Default Constructor:
- Definition: If a class does not explicitly define any constructors, Java automatically provides a default constructor. It initializes object fields with default values.
- Syntax:
public ClassName() {
// Default constructor body
}
b. Parameterized Constructor:
- Definition: A parameterized constructor allows you to initialize object fields with specific values passed as arguments during object creation.
- Syntax:
public ClassName(parameter1Type parameter1, parameter2Type parameter2, ...) {
// Parameterized constructor body
// Initialization code using parameters
}
public class Car {
// Data members
private String brand;
private String model;
private int year;
// Default constructor
public Car() {
this.brand = "Unknown";
this.model = "Unknown";
this.year = 0;
}
// Parameterized constructor
public Car(String brand, String model, int year) {
this.brand = brand;
this.model = model;
this.year = year;
}
// Method to display car details
public void displayDetails() {
System.out.println("Brand: " + brand);
System.out.println("Model: " + model);
System.out.println("Year: " + year);
}
}
public class Main {
public static void main(String[] args) {
// Creating objects using constructors
Car defaultCar = new Car(); // Invokes default constructor
Car paramCar = new Car("Toyota", "Camry", 2021); // Invokes parameterized constructor
// Displaying details of cars
System.out.println("Default Car Details:");
defaultCar.displayDetails();
System.out.println("\nParameterized Car Details:");
paramCar.displayDetails();
}
}
- Initialization: Constructors initialize object fields and prepare them for use.
- Object Creation: Constructors are automatically invoked when objects are created using the
new
keyword.
- Overloading: Like methods, constructors can be overloaded to provide multiple ways of initializing objects.
- Chaining: Constructors can call other constructors within the same class using
this()
or super()
.
Best Practices:
- Always provide a no-argument default constructor, especially if you define parameterized constructors.
- Use constructors to ensure object integrity and to enforce mandatory initialization.
Conclusion:
Constructors are fundamental components of Java classes, responsible for initializing object state during object creation. By understanding and utilizing constructors effectively, Java developers can ensure proper object initialization and maintain code consistency.
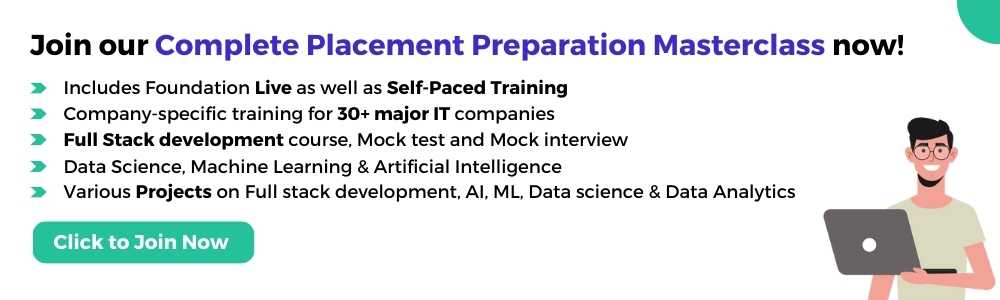
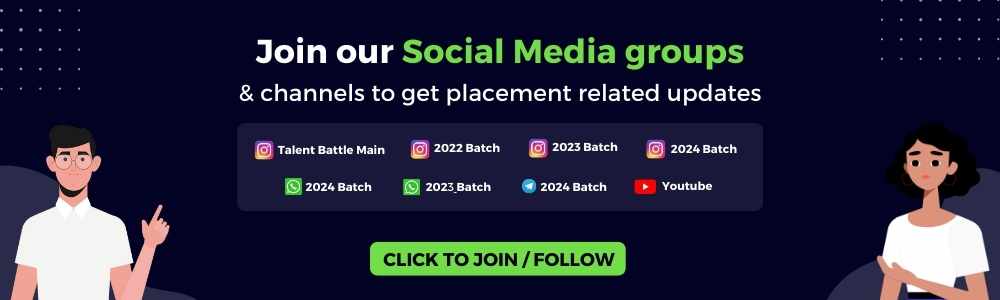