Table of Contents (Click any Topic to view first)
- Classes and Objects
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
- Association, Aggregation, and Composition
Understanding the Object Oriented Programming (OOP's Concepts) in Java
Java is a versatile programming language that supports object-oriented programming (OOP) principles. Understanding OOP concepts is crucial for building robust, scalable, and maintainable Java applications. Let's delve into the core OOP concepts in Java with detailed explanations and examples.
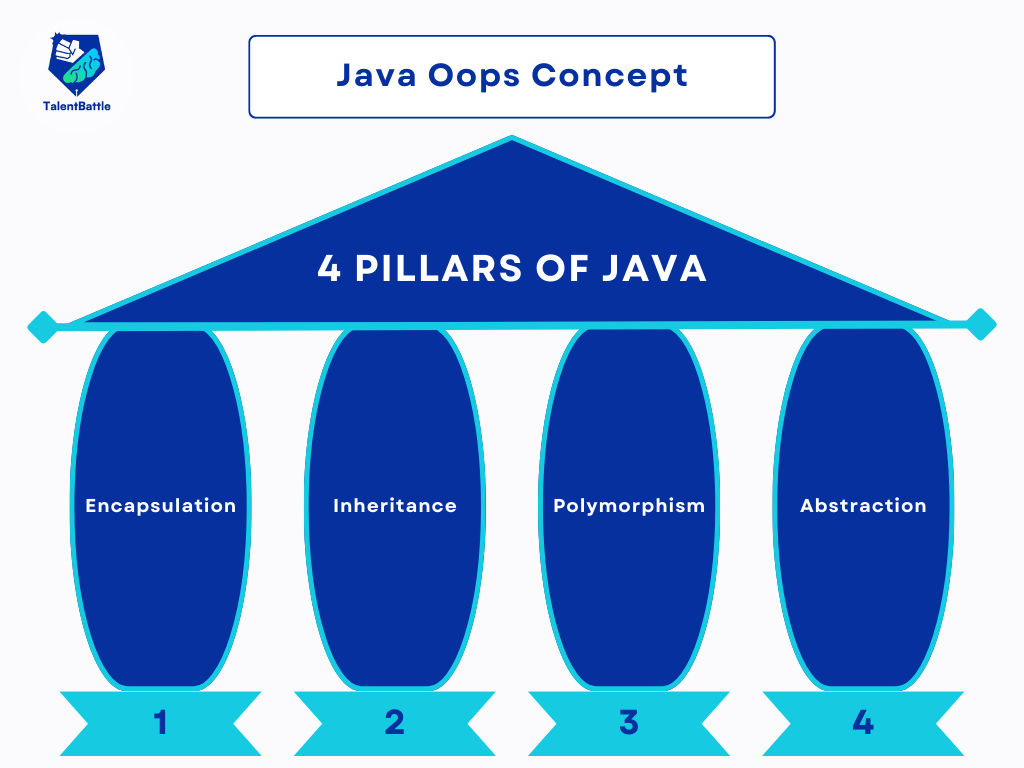
Classes:
- Definition: A class is a blueprint or template for creating objects. It encapsulates data for the object and defines methods to operate on that data.
- Syntax:
public class ClassName {
// Data members (fields)
// Constructor(s)
// Methods
}
Objects:
- Definition: An object is an instance of a class. It represents a real-world entity and provides a way to access the methods and data members defined in its class.
- Syntax:
ClassName objectName = new ClassName();
- Definition: Encapsulation is the bundling of data (attributes) and methods (behaviors) that operate on the data within a single unit or class. It hides the internal state of objects and restricts direct access to them.
- Example:
public class Car {
private String model;
private int year;
// Getters and setters for model and year
// Other methods
}
- Definition: Inheritance is a mechanism by which one class (subclass or child class) inherits properties and behaviors from another class (superclass or parent class). It promotes code reuse and establishes a hierarchical relationship between classes.
- Example:
public class Animal {
public void eat() {
System.out.println("Animal is eating");
}
}
public class Dog extends Animal {
// Inherits the eat() method from Animal class
}
- Definition: Polymorphism allows objects to be treated as instances of their superclass. It enables methods to be called on objects of different classes through a common interface, leading to flexibility and extensibility in code.
- Example:
public interface Animal {
void makeSound();
}
public class Dog implements Animal {
public void makeSound() {
System.out.println("Woof!");
}
}
public class Cat implements Animal {
public void makeSound() {
System.out.println("Meow!");
}
}
5. Abstraction:
- Definition: Abstraction involves hiding complex implementation details and exposing only the essential features of an object. It allows programmers to focus on what an object does rather than how it does it.
- Example:
public abstract class Shape {
abstract double area();
}
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double area() {
return Math.PI * radius * radius;
}
}
- Association: Represents a relationship between two or more classes where each class has its own lifecycle, and there is no owner.
- Aggregation: Represents a "has-a" relationship between classes where one class contains references to another class but does not own it.
- Composition: Represents a strong "contains-a" relationship between classes where one class owns another class and is responsible for its lifetime.
Conclusion:
Understanding and applying these core OOP concepts in Java is essential for writing efficient, maintainable, and scalable code. By leveraging encapsulation, inheritance, polymorphism, abstraction, and relationships like association, aggregation, and composition, Java developers can build robust and flexible software solutions.
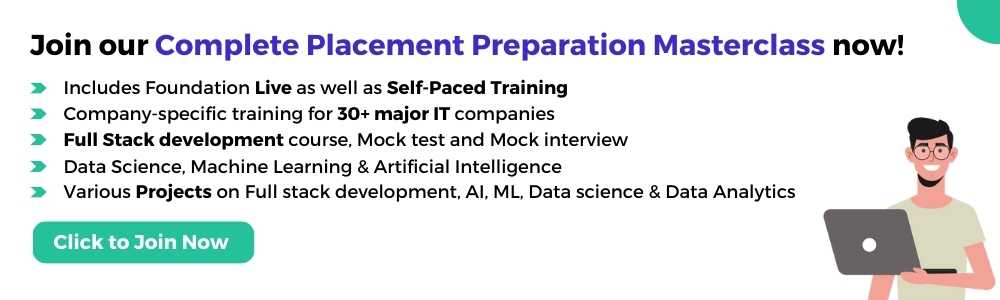
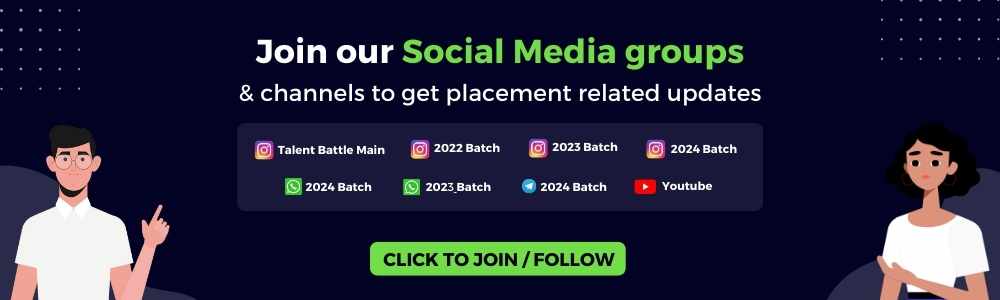