Table of Contents (Click any Topic to view first)
- What is the Scanner Class?
- How Does the Scanner Class Work?
- Example Usage of the Scanner Class
Are you ready to dive into the world of user input in Java? Say hello to the Scanner class! In this guide, we'll take a beginner-friendly look at what the Scanner class is, how it works, and how you can use it to interact with your Java programs.
The Scanner class in Java is a part of the java.util package that allows you to read input from various sources like the keyboard or a file. It provides methods for parsing and tokenizing input into different data types, such as integers, floats, and strings.
Using the Scanner class involves a few simple steps:
- Import the Scanner Class: Before using the Scanner class, you need to import it into your Java program. You can do this by including the following line at the beginning of your code:
import java.util.Scanner;
- Create a Scanner Object: Next, you need to create a Scanner object to read input. You can create a Scanner object by specifying the input source, such as System.in for keyboard input or a file input stream for reading from a file.
Scanner scanner = new Scanner(System.in);
- Read Input: Once you have a Scanner object, you can use its methods to read input. The most commonly used method is nextLine(), which reads a line of text entered by the user.
String input = scanner.nextLine();
- Parse Input: After reading input, you can parse it into different data types using methods like nextInt(), nextFloat(), or nextBoolean().
int number = scanner.nextInt();
- Close the Scanner: It's good practice to close the Scanner object once you're done with it to release system resources.
scanner.close();
Let's look at a simple example to understand how the Scanner class works:
import java.util.Scanner;
public class InputExample {
public static void main(String[] args) {
// Create a Scanner object for user input
Scanner scanner = new Scanner(System.in);
// Prompt the user to enter their name
System.out.print("Enter your name: ");
// Read the user's input
String name = scanner.nextLine();
// Prompt the user to enter their age
System.out.print("Enter your age: ");
// Read the user's input as an integer
int age = scanner.nextInt();
// Display the user's name and age
System.out.println("Hello, " + name + "! You are " + age + " years old.");
// Close the Scanner object
scanner.close();
}
}
Conclusion
The Scanner class in Java provides a convenient way to read input from users, making your programs interactive and dynamic. By following the steps outlined in this guide, you can easily incorporate user input into your Java applications. So go ahead, experiment with the Scanner class, and unlock new possibilities for your Java projects!
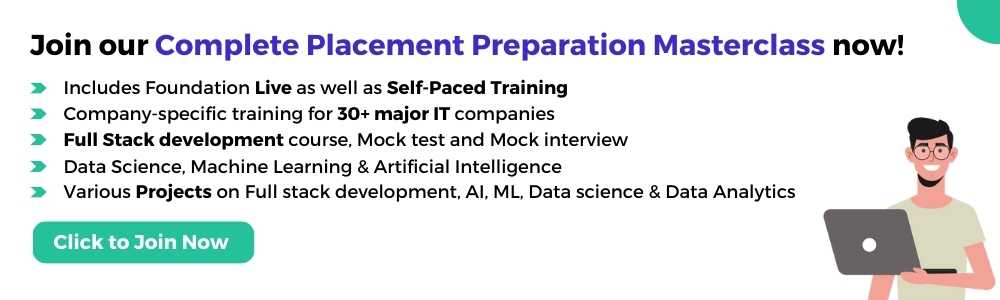
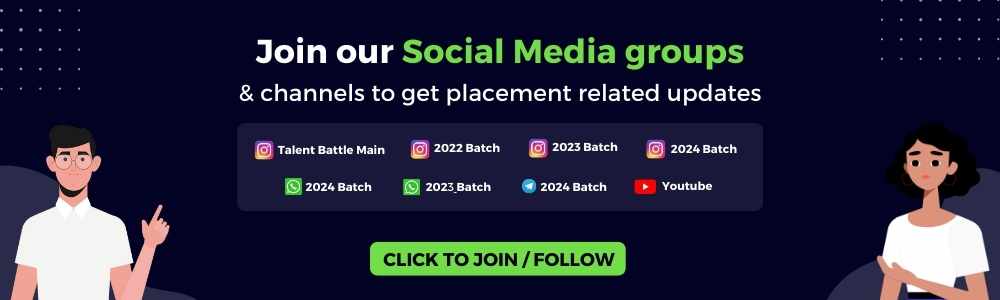