Table of Contents (Click any Topic to view first)
- Definition of the static Keyword
- Usage Scenarios
- Example: Demonstrating the Usage of static in Java
Understanding the Static Keyword in Java
In Java programming, the static
keyword is a powerful tool that allows for the creation of class members and methods that belong to the class itself, rather than individual instances of the class. It plays a crucial role in defining class-level behavior and data access. Let's explore the static
keyword in Java, its usage, and its implications.
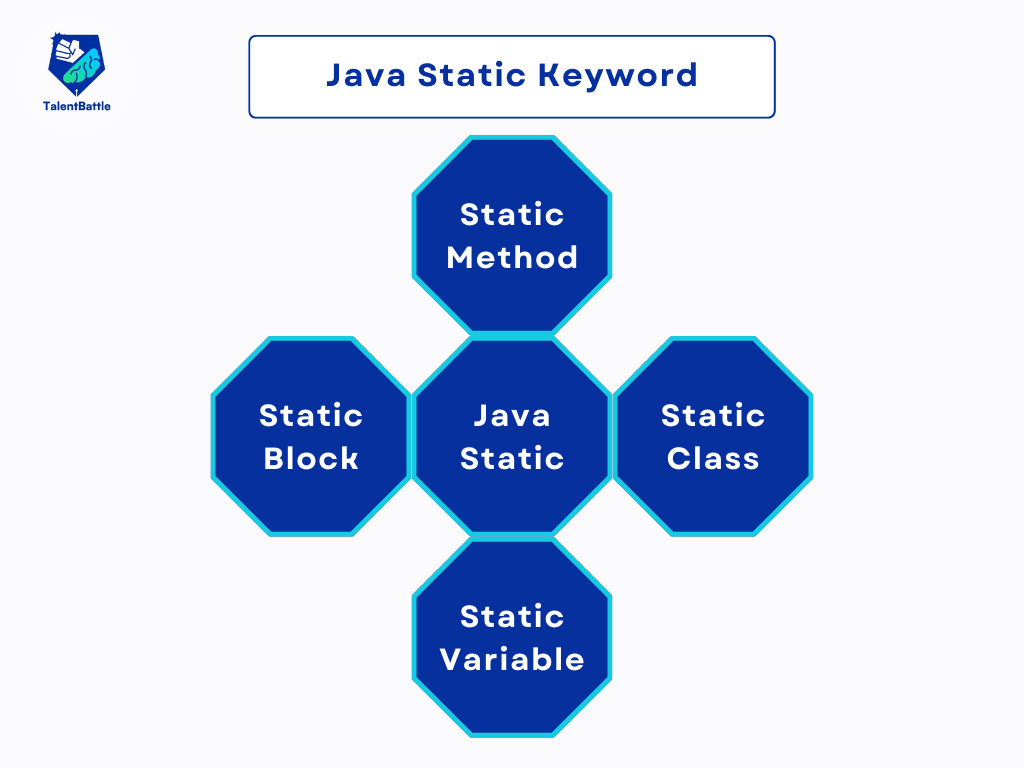
- Definition: The
static
keyword in Java is used to create class-level variables and methods. These members are shared among all instances of the class and can be accessed directly through the class name, without the need to create an object of the class.
- Syntax:
- For Variables:
static dataType variableName;
- For Methods:
static returnType methodName(parameters) { }
a. Static Variables (Class Variables):
- Definition: Static variables are shared among all instances of the class. They are initialized only once at the start of the program execution.
- Usage:
public class MyClass {
static int count = 0; // Static variable
}
b. Static Methods (Class Methods):
- Definition: Static methods belong to the class rather than individual objects. They can be called using the class name and are commonly used for utility methods.
- Usage:
public class MyClass {
static void display() { // Static method
System.out.println("Hello, Java!");
}
}
public class MyClass {
static int count = 0; // Static variable
// Static method to increment count
static void incrementCount() {
count++;
}
// Static method to display count
static void displayCount() {
System.out.println("Count: " + count);
}
}
public class Main {
public static void main(String[] args) {
// Accessing static variable and methods without creating object
MyClass.incrementCount();
MyClass.displayCount();
}
}
Key Concepts:
- Shared State: Static members are shared among all instances of the class and maintain a single state across the class.
- Class-Level Access: Static members can be accessed directly through the class name, making them accessible without creating an object of the class.
- Memory Efficiency: Static variables are allocated memory once and exist throughout the program's execution.
Best Practices:
- Use static variables and methods when the behavior or data is common across all instances of the class.
- Avoid excessive use of static members, as they can lead to tight coupling and reduce flexibility.
Conclusion:
The static
keyword in Java enables the creation of class-level variables and methods, providing shared behavior and data across all instances of the class. By understanding its usage and implications, Java developers can design efficient and maintainable code.
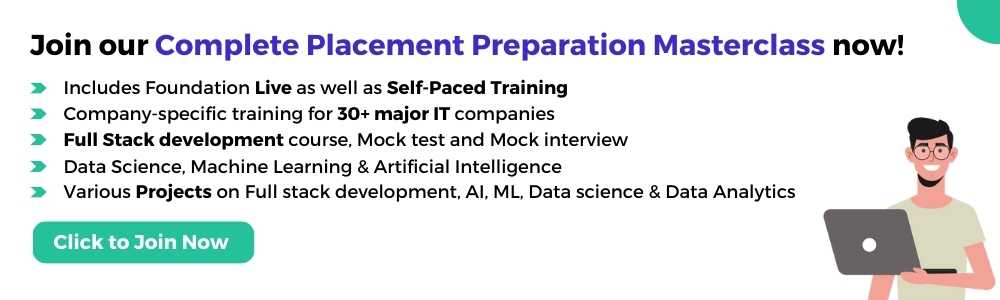
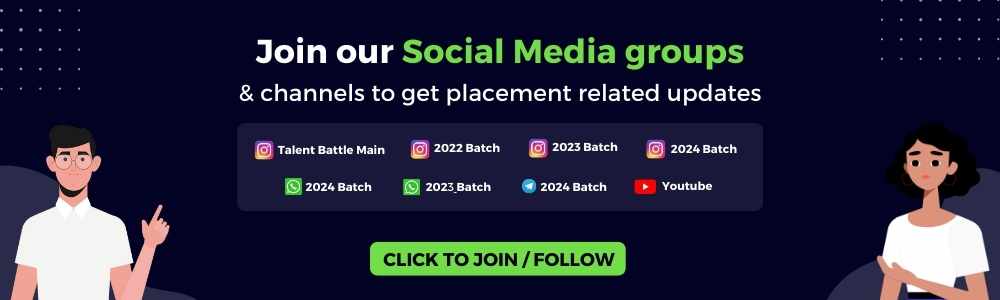