Table of Contents (Click any Topic to view first)
- Understanding the Super Keyword in Java
- Using super to Refer to Parent Class Instance Variable
- Using super to Invoke Parent Class Method
- Using super to Invoke Parent Class Constructor
- Real Use of super Keyword
The super
keyword in Java is a reference variable used to refer to the immediate parent class object. It is primarily used in inheritance to access members of the parent class from the child class. The super
keyword provides three main functionalities:
- Referencing the parent class's instance variable.
- Invoking the parent class's method.
- Invoking the parent class's constructor.
When parent and child classes have a common field, the super
keyword can be used to access the parent class's field.
Example:
class Animal {
String color = "white";
}
class Dog extends Animal {
String color = "black";
void printColor() {
System.out.println(color); // prints color of Dog class
System.out.println(super.color); // prints color of Animal class
}
}
class TestSuper1 {
public static void main(String args[]) {
Dog d = new Dog();
d.printColor();
}
}
Output:
black
white
In this example, the color
field is defined in both the Animal
and Dog
classes. Using super.color
in the Dog
class's printColor
method accesses the color
field of the Animal
class.
The super
keyword can also be used to call the parent class's method from the child class, which is useful when the method is overridden.
Example:
class Animal {
void eat() {
System.out.println("eating...");
}
}
class Dog extends Animal {
void eat() {
System.out.println("eating bread...");
}
void bark() {
System.out.println("barking...");
}
void work() {
super.eat();
bark();
}
}
class TestSuper2 {
public static void main(String args[]) {
Dog d = new Dog();
d.work();
}
}
Output:
eating...
barking...
In this example, the eat
method is overridden in the Dog
class. Using super.eat()
calls the eat
method of the Animal
class.
The super
keyword can also be used to invoke the parent class's constructor, which is necessary when you want to initialize the parent class's properties from the child class.
Example:
class Animal {
Animal() {
System.out.println("animal is created");
}
}
class Dog extends Animal {
Dog() {
super();
System.out.println("dog is created");
}
}
class TestSuper3 {
public static void main(String args[]) {
Dog d = new Dog();
}
}
Output:
animal is created
dog is created
In this example, the super()
call in the Dog
class's constructor invokes the Animal
class's constructor.
The super
keyword is often used to initialize properties inherited from a parent class.
Example:
class Person {
int id;
String name;
Person(int id, String name) {
this.id = id;
this.name = name;
}
}
class Emp extends Person {
float salary;
Emp(int id, String name, float salary) {
super(id, name); // reusing parent constructor
this.salary = salary;
}
void display() {
System.out.println(id + " " + name + " " + salary);
}
}
class TestSuper5 {
public static void main(String[] args) {
Emp e1 = new Emp(1, "ankit", 45000f);
e1.display();
}
}
Output:
1 ankit 45000
In this example, the Emp
class reuses the Person
class's constructor using super(id, name)
to initialize the id
and name
fields, while Emp
's constructor initializes the salary
field.
Summary
The super
keyword in Java is a powerful tool for managing inheritance, allowing for:
- Accessing parent class fields and methods.
- Invoking parent class constructors to initialize inherited properties. By using
super
, you can create clear and maintainable code that leverages the full power of inheritance.
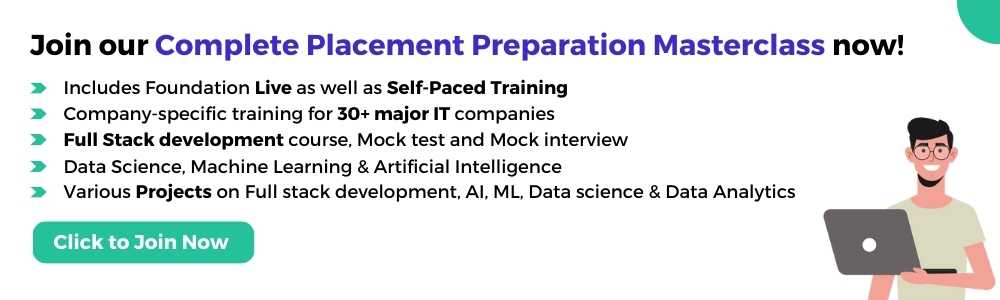
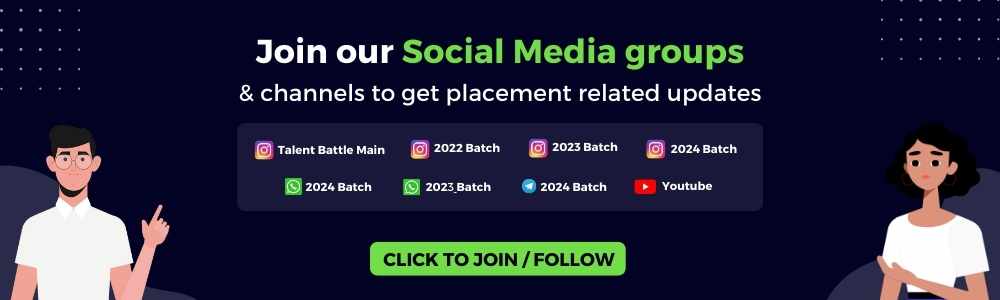