Table of Contents (Click any Topic to view first)
- Definition of the 'this' Keyword
- Referring to Current Class Instance Variables
- Invoking Current Class Methods
- Invoking Current Class Constructors
- Passing 'this' as an Argument in Method Calls
- Passing 'this' as an Argument in Constructor Calls
- Returning the Current Class Instance
Understanding the This Keyword in Java
In Java programming, the 'this' keyword plays a crucial role in referring to the current instance of a class. It is used to access instance variables, methods, and constructors within the same class. Understanding how 'this' works is essential for writing clean and readable code. Let's explore the 'this' keyword in Java, its usage, and its significance.
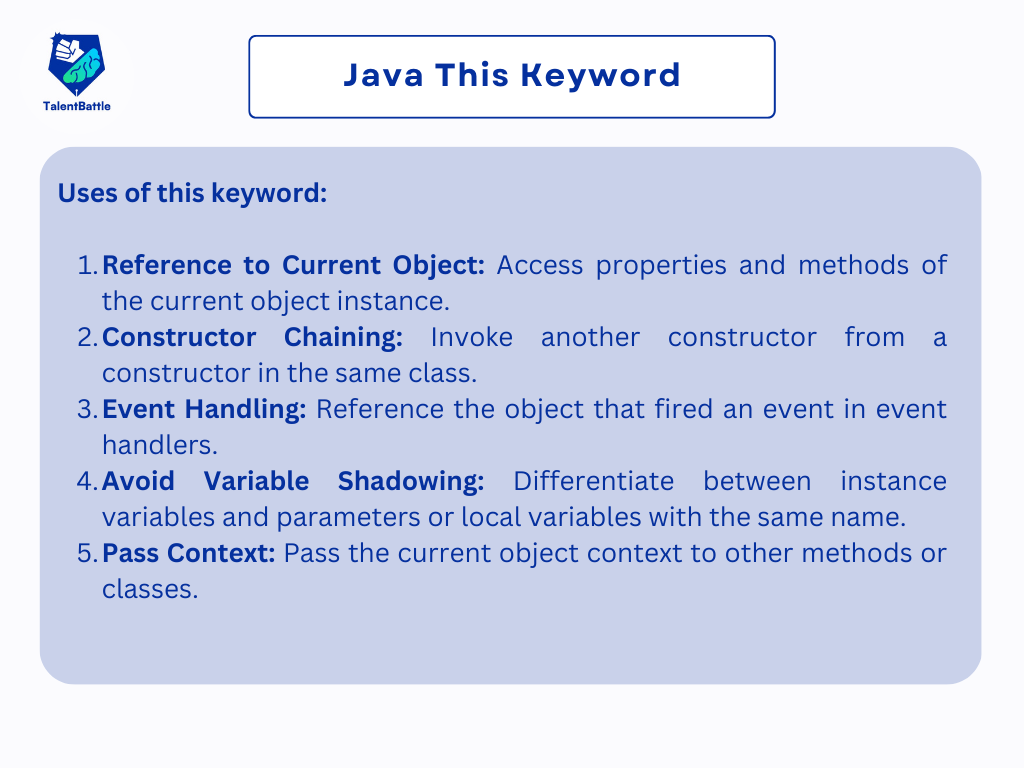
- Definition: The 'this' keyword in Java refers to the current object or instance of a class. It can be used to access instance variables and methods, differentiate between instance and local variables, and invoke one constructor from another constructor within the same class.
- Syntax:
- To access instance variables:
this.variableName
- To invoke methods:
this.methodName()
- To invoke constructors:
this(parameters)
The this
keyword can resolve ambiguity between instance variables and parameters with the same name.
Without the this
Keyword
Consider the following example where the parameters and instance variables share the same names:
class Student {
int rollno;
String name;
float fee;
Student(int rollno, String name, float fee) {
rollno = rollno;
name = name;
fee = fee;
}
void display() {
System.out.println(rollno + " " + name + " " + fee);
}
}
class TestThis1 {
public static void main(String args[]) {
Student s1 = new Student(111, "ankit", 5000f);
Student s2 = new Student(112, "sumit", 6000f);
s1.display();
s2.display();
}
}
Output:
0 null 0.0
0 null 0.0
Here, the assignments don't work as intended because the parameters overshadow the instance variables.
Using the this
Keyword
class Student {
int rollno;
String name;
float fee;
Student(int rollno, String name, float fee) {
this.rollno = rollno;
this.name = name;
this.fee = fee;
}
void display() {
System.out.println(rollno + " " + name + " " + fee);
}
}
class TestThis2 {
public static void main(String args[]) {
Student s1 = new Student(111, "ankit", 5000f);
Student s2 = new Student(112, "sumit", 6000f);
s1.display();
s2.display();
}
}
Output:
111 ankit 5000.0
112 sumit 6000.0
In this example, the this
keyword differentiates between instance variables and parameters, ensuring proper assignment.
The this
keyword can invoke methods within the same class.
class A {
void m() {
System.out.println("hello m");
}
void n() {
System.out.println("hello n");
this.m(); // Explicit use of this
}
}
class TestThis4 {
public static void main(String args[]) {
A a = new A();
a.n();
}
}
Output:
hello n
hello m
The this()
constructor call invokes the current class constructor, facilitating constructor chaining.
Calling Default Constructor from Parameterized Constructor
class A {
A() {
System.out.println("hello a");
}
A(int x) {
this();
System.out.println(x);
}
}
class TestThis5 {
public static void main(String args[]) {
A a = new A(10);
}
}
Output:
hello a
10
Calling Parameterized Constructor from Default Constructor
class A {
A() {
this(5);
System.out.println("hello a");
}
A(int x) {
System.out.println(x);
}
}
class TestThis6 {
public static void main(String args[]) {
A a = new A();
}
}
Output:
5
hello a
You can pass this
as an argument to methods to reference the current object.
class S2 {
void m(S2 obj) {
System.out.println("method is invoked");
}
void p() {
m(this);
}
public static void main(String args[]) {
S2 s1 = new S2();
s1.p();
}
}
Output:
method is invoked
Passing this
in constructor calls can be useful for using one object across multiple classes.
class B {
A4 obj;
B(A4 obj) {
this.obj = obj;
}
void display() {
System.out.println(obj.data);
}
}
class A4 {
int data = 10;
A4() {
B b = new B(this);
b.display();
}
public static void main(String args[]) {
A4 a = new A4();
}
}
Output:
10
You can return the current class instance using this
keyword.
class A {
A getA() {
return this;
}
void msg() {
System.out.println("Hello java");
}
}
class Test1 {
public static void main(String args[]) {
new A().getA().msg();
}
}
Output:
Hello java
This program demonstrates that this
refers to the current class instance. Both the reference variable and this
will print the same reference ID.
class A5 {
void m() {
System.out.println(this);
}
public static void main(String args[]) {
A5 obj = new A5();
System.out.println(obj);
obj.m();
}
}
Output:
A5@<reference_ID>
A5@<reference_ID>
Using the this
keyword effectively can improve the clarity and maintainability of your Java code, ensuring that your references to the current instance are clear and unambiguous.
Conclusion:
The 'this' keyword in Java is a powerful tool for referring to the current instance of a class, enabling access to instance variables, methods, and constructors. By understanding its usage and significance, Java developers can write cleaner and more maintainable code.
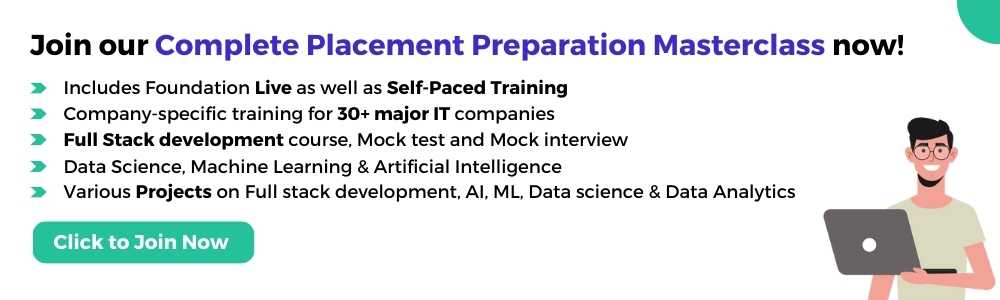
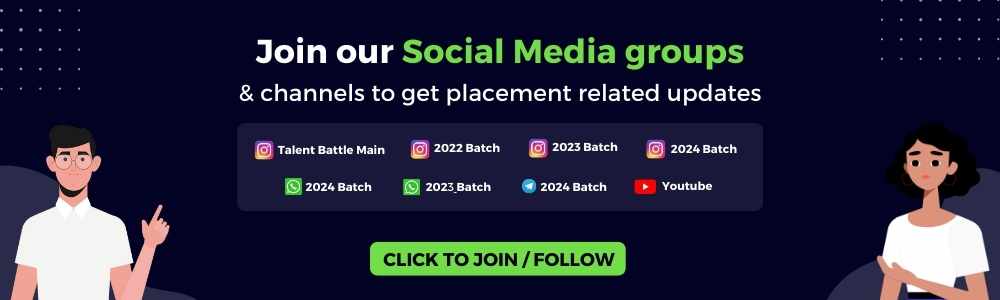