Table of Contents (Click any Topic to view first)
1. Variables in Java
2. Types of Variables in Java
3. Variable Declaration and Initialization
4. Variable Scope
5. Final Variables
Variables are fundamental to any programming language, and Java is no exception. They are used to store data that can be manipulated and retrieved throughout the program. In Java, variables have specific types that determine the kind of data they can hold, such as integers, floating-point numbers, characters, and more. This blog will explore the different types of variables in Java, their declaration, initialization, and scope.
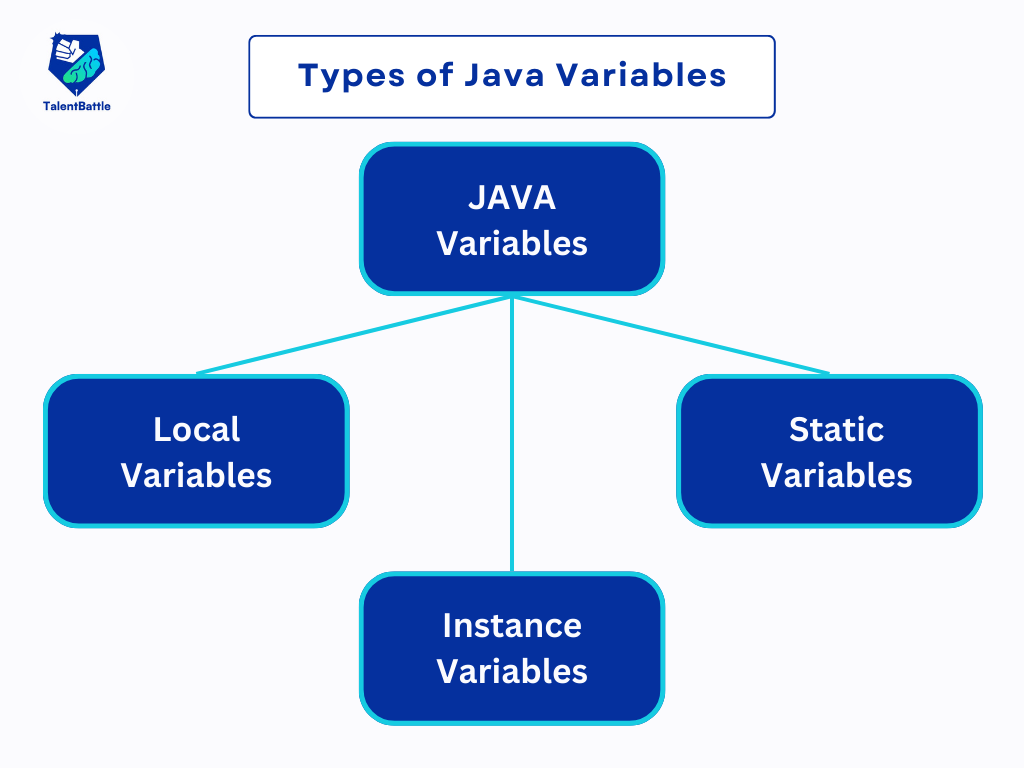
- Local Variables
- Definition: Variables declared inside a method, constructor, or block.
- Scope: Only accessible within the method, constructor, or block where they are declared.
- Initialization: Must be initialized before use.
- Example:
public void myMethod() {
int localVar = 10; // local variable
System.out.println(localVar);
}
- Instance Variables
- Definition: Variables declared inside a class but outside any method, constructor, or block.
- Scope: Accessible from any method, constructor, or block within the class.
- Initialization: Initialized to default values if not explicitly initialized (0 for numeric types, null for object references, etc.).
- Example:
public class MyClass {
int instanceVar; // instance variable
public void display() {
System.out.println(instanceVar);
}
}
- Class/Static Variables
- Definition: Variables declared with the static keyword inside a class but outside any method, constructor, or block.
- Scope: Accessible from any method, constructor, or block within the class and can be accessed directly using the class name.
- Initialization: Initialized to default values if not explicitly initialized.
- Example:
public class MyClass {
static int staticVar; // static variable
public static void display() {
System.out.println(staticVar);
}
}
- Declaration: Specifies the type and name of the variable.
int myVar;
- Initialization: Assigns a value to the variable.
myVar = 25;
- Combined Declaration and Initialization:
int myVar = 25;
Java supports various data types for variables, categorized into primitive data types and reference data types.
- Primitive Data Types:
- byte, short, int, long (for integers)
- float, double (for floating-point numbers)
- char (for characters)
- boolean (for true/false values)
Example:
int age = 30;
double salary = 85000.50;
char grade = 'A';
boolean isActive = true;
- Reference Data Types:
- Used to refer to objects.
- Examples include class types, interface types, array types.
Example:
String name = "John Doe";
int[] numbers = {1, 2, 3, 4, 5};
- Local Scope: Variables declared inside a method are only accessible within that method.
- Instance Scope: Variables declared inside a class but outside any method are accessible by all methods of the class.
- Class Scope: Static variables are shared among all instances of a class and can be accessed using the class name.
- Definition: Variables declared with the final keyword cannot be changed once initialized.
- Usage: Used to create constants.
Example:
final int MAX_AGE = 100;
Understanding variables in Java is crucial for effective programming. They are the building blocks that store data values and have a specific type, scope, and lifetime. By mastering variable declaration, initialization, and scope, you can write more efficient and error-free Java programs. Happy coding!
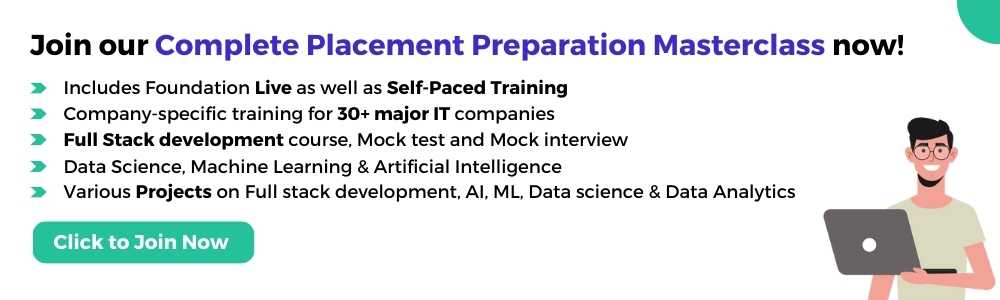
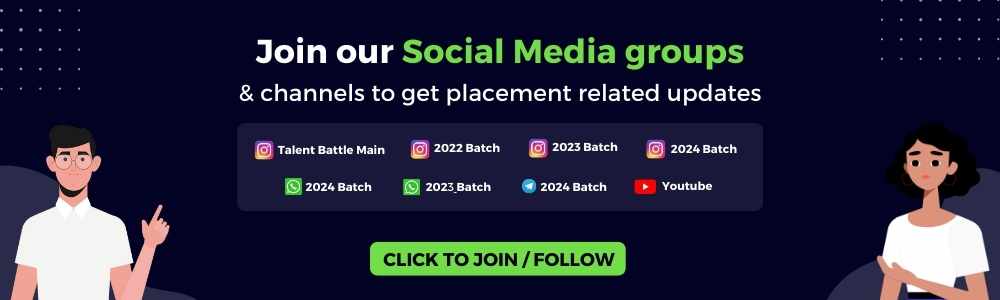