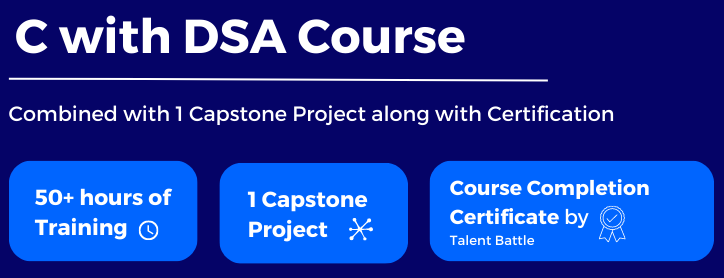
Course Overview:
Data Structures and Algorithms are very important when it comes to programming/coding rounds during campus and off-campus placements. Service-Based or Product-based companies both specifically focus more on knowledge of Data Structures and Algorithms. It is very important when it comes to code implementation. This Data Structures & Algorithms Recorded Training will help all stream students to learn DSA programming right from the basics and help them crack programming rounds for all major companies.
This Self-Paced C Programming with Data Structures & Algorithms will help all stream students to learn C programming & DSA right from the basics and help them crack programming rounds for all major companies.
C programming language is a mother of all programming languages.
Who Should Take this training?
All the stream students can take this training to learn to program from the basics which will help crack programming rounds conducted by various companies.
Talent Battle Certification
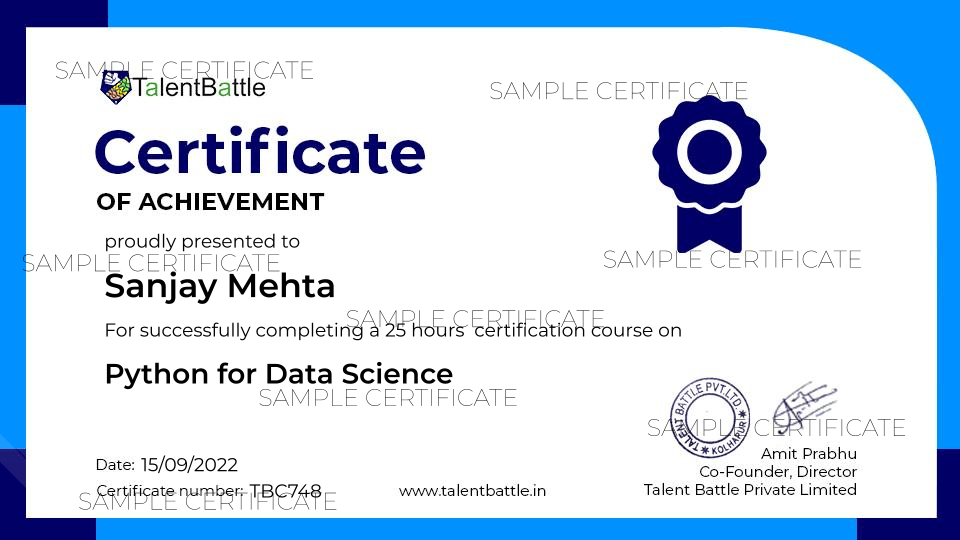
Features
C training from basics to advanced level
|
Project along with Certification
|
Mentorship/Doubt solving
We stand behind our training and service, and we want you to achieve your target with complete Doubt clarification and mentor support throughout the preparation. Provision of Private Telegram group for quick and direct doubt resolutions from Trainers.
|
Validity & Access
The course can be accessed from Mobile/Laptop/Desktop/Tablet. There will be a 6 months validity for this training.
|
Course Contents
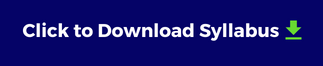
For Doubts Contact Us on Whatsapp: +91-8999359336 or Email us at contact@talentbattle.in
Course Creators & Mentors
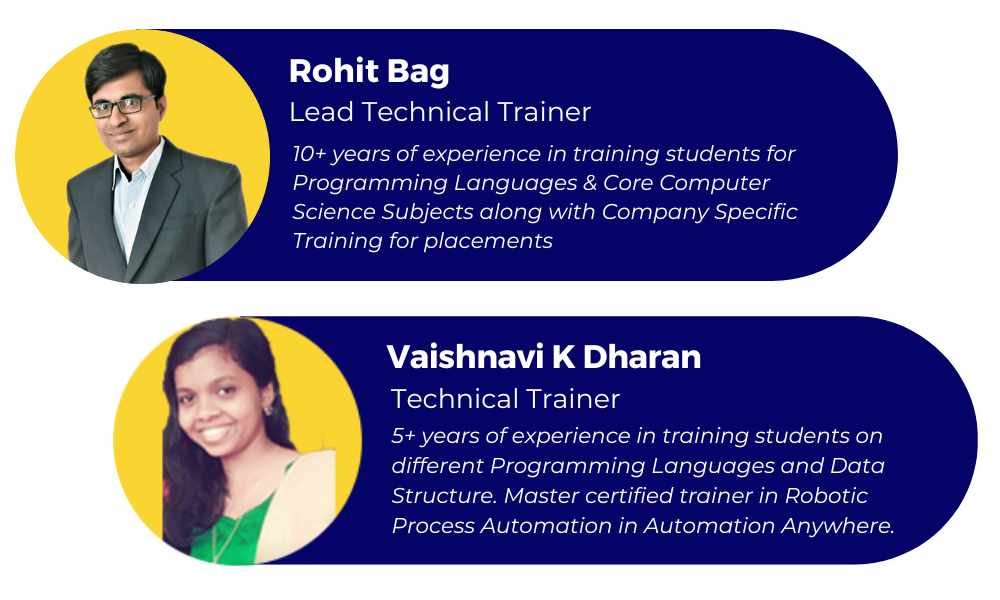